This combines two bar charts as set out in the question.
library(tibble)
library(dplyr)
library(tidyr)
library(ggplot2)
tib <-
tibble(t1 = table(test),
t2 = table(test2)) %>%
mutate(group = 1:4)
ggplot()+
geom_bar(data = select(tib, -2), aes(group, t1), stat = "identity", fill = "red", alpha = 0.25, width = 1)+
geom_bar(data = select(tib, -1), aes(group, t2), stat = "identity", fill = "green", alpha = 0.25,width = 1)
Which results in:
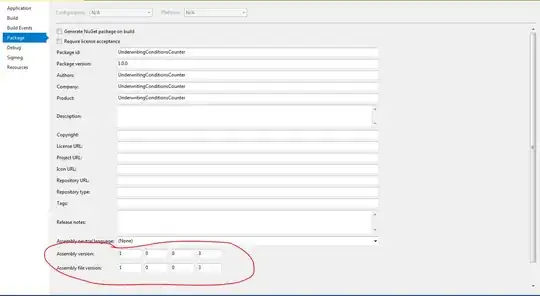
However, what I think you might be really looking for is:
library(tibble)
library(dplyr)
library(tidyr)
library(ggplot2)
set.seed(85)
test <- replicate(10,sample(0:3,5,rep=TRUE))
set.seed(108)
test2 <- replicate(10,sample(0:3,5,rep=TRUE))
tib1(tibble)
tib1 <-
tibble(t1 = c(test),
t2 = c(test2)) %>%
pivot_longer(cols = c(t1, t2), names_to = "test", values_to = "score")
ggplot(tib1, aes(score, fill = test))+
geom_histogram(bins = 4, alpha = 0.5, position = "identity")
Which gives you:
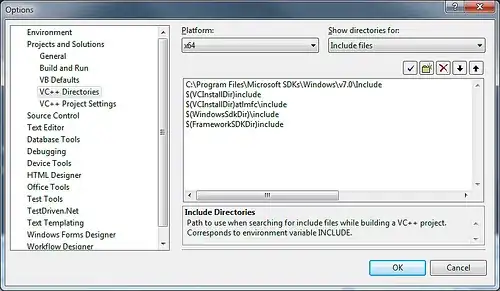
If the number of observations for each test are different and you want to plot a histogram: create two dataframes and combine them for graphing
set.seed(85)
test1 <- tibble(score = c(replicate(20, sample(0:3, 5, rep = TRUE))),
test = "t1")
set.seed(108)
test2 <- tibble(score = c(replicate(10, sample(0:3, 5, rep = TRUE))),
test = "t2")
tib1 <-
test1 %>%
bind_rows(test2)
ggplot(tib1, aes(score, fill = test))+
geom_histogram(bins = 4, alpha = 0.5, position = "identity")
If you prefer the geom_bar version you can adapt the previous code as follows:
ggplot()+
geom_bar(data = test1, aes(score), stat = "count", fill = "red", alpha = 0.25, width = 1)+
geom_bar(data = test2, aes(score), stat = "count", fill = "green", alpha = 0.25,width = 1)
By the way you could probably simplify your code, unless you have other reasons for using replicate as: c(replicate(10, sample(0:3, 5, rep = TRUE))) == sample(0:3, 50, rep = TRUE)
set.seed(108)
s1 <- c(replicate(10, sample(0:3, 5, rep = TRUE)))
set.seed(108)
s2 <- sample(0:3, 50, rep = TRUE)
tib <-
tibble(t1 = s1,
t2 = s2) %>%
pivot_longer(t1:t2, names_to = "test", values_to = "score")
ggplot(tib, aes(score, fill = test))+
geom_histogram(bins = 4, alpha = 0.5, position = "identity") +
facet_wrap(~test)