A sample example that demonstrates usage of Math.Round() function:
using System;
class MainClass {
public static void Main (string[] args) {
double result = 0.3937947494;
Console.WriteLine(Math.Round(result,3));
}
}
// here result is = 0.3937947494, but you will get output as 0.394 in the console.
VERSION 2:
using System;
public class Program
{
public static void Main()
{
int hits = 165;
int atBats = 419;
double result = (double)hits / (double)atBats;
Console.WriteLine("Your batting average is: " +Math.Round(result,3));
}
}
// this produces the same result
// Your batting average is: 0.394
Screenshot for second alternative:

VERSION 3: Serves better readability (as suggested correctly by @Manti_Core
using System;
public class Program
{
public static void Main()
{
double hits = 165;
double atBats = 419;
double result = hits/atBats;
Console.WriteLine("Your batting average is: " + (hits / atBats).ToString("0.000"));
}
}
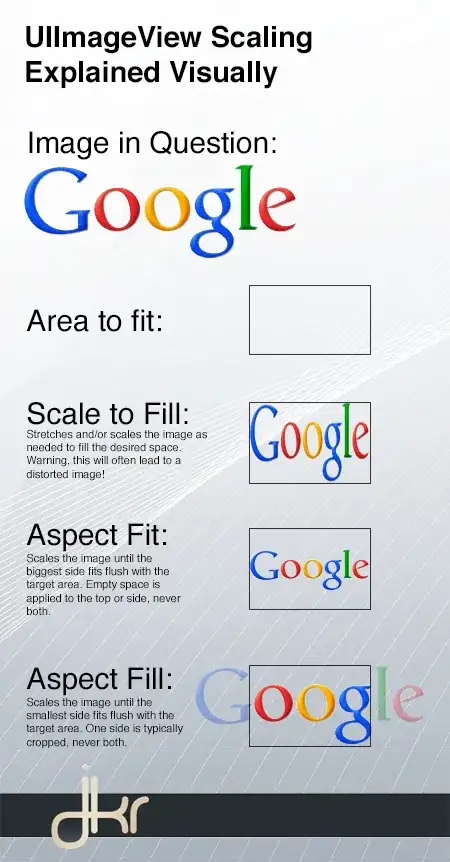
Hope this helps.