I am trying to call a function calcAge()
inside a javascript object. calcAge()
function required a parameter birthYr. If i use calcAge(1998)
this is working. But i want this birthYr parameter will be the own element of that object, But this is Showing Undefined. How can i do this? and also i can i get person object retiredIn element with the returned value of that function? why person object always showing retiredIn element as a Function? i want to get it as a function output. How to do this?
I Try,
function calcAge(birthYr) {
return 2020 - birthYr;
}
var person = {
birthYear: 1998,
age: calcAge(this.birthYear), //This is Showing Undefined
retiredIn: function () {
return 65 - this.age; //So this returns a NaN
},
name: "Monir",
job: "Teacher"
};
console.log(person.birthYear); //This is Ok when i am trying to call it from outside!
console.log(person);
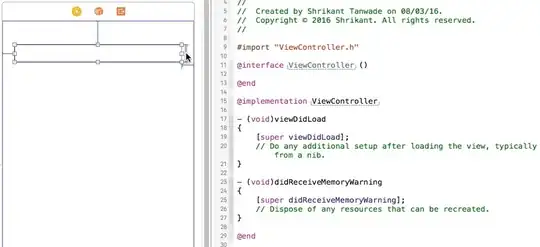