To elaborate on Avoid using Select.
Using methods like Select
, Copy
and Paste
are easy to read and visualise what your code is doing - but - it comes at a cost. It's not the most efficient performing code which can really show once your applications start getting bigger and bigger.
Big or small, it's a good practice to avoid these kinds of methods and refer directly to your Worksheet
object and relevant Range
on the worksheet to find data and put it somewhere else.
To paraphrase the top answers on that question, here are the main reasons to avoid these methods;
- Select and Selection are a common cause for run-time errors.
- This is because it relies on nothing (either code or the user) changing the sheets focus, If someone clicks somewhere else or something in your code changes the sheet or selection, it
can will cause funky results or an error.
- As mentioned above, they slow your code down. With a bit of googling you'll find tests performed showing the differences in time between using these methods and avoiding them.
with that said, this should resolve your issue:
Sub CopyPaste3()
Dim row As Integer
Dim lastrow As Integer
Dim r As Integer
Dim S1 As Worksheet
Dim S2 As Worksheet
Dim myArray As Variant
Dim Destination As Range
Set S1 = Worksheets("Sheet1")
Set S2 = Worksheets("sheet2")
rowg = 12
lastrow = S1.Range("A" & Rows.Count).End(xlUp).row
For r = 2 To lastrow
If S1.Range("D" & r).Value = "Y" Then
myArray = S1.Range("E" & r & ":F" & r).Value
Set Destination = S2.Cells(rowg, 7)
Set Destination = Destination.Resize(UBound(myArray, 2), 1)
Destination = Application.Transpose(myArray)
rowg = S2.Range("G" & Rows.Count).End(xlUp).row + 1
End If
Next r
End Sub
I've made use of an array to grab the required column E to F values and transpose those to your destination range. I've used the rowg
variable that you are updating with each iteration when the data is moved - because you had hardcoded 12 as the row, it was previously overwriting your values each time rather than moving to the next blank row.
Here are snips of sample sheet1 and sheet2 data:
Sheet1
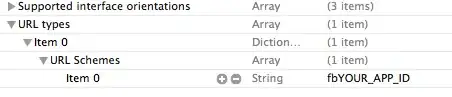
Sheet2:
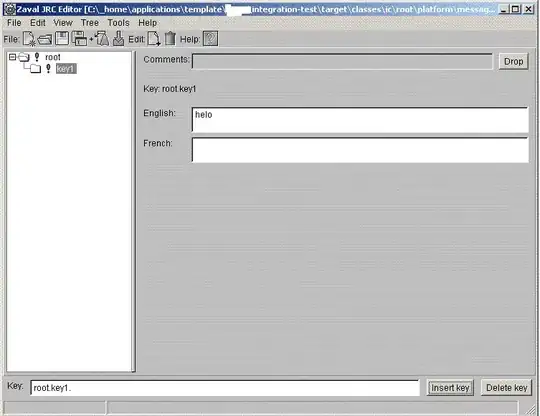