This IS the correct way to set multiple headers for 1 request:
<?php
if(isset($_GET['submit'])) {
header("Location:Page2.php");
header("Refresh:0");
}
?>
Note: In this sample we are redirecting to Page2.php
due to that the Refresh:0
is useless!
Saying useless does not mean that Page1.php
will not output the header: Refresh:0
.
NEVER USE: Location
+ Refresh
for same request unless you know what you are doing.
Page1 will output both headers but you will not notice any difference from a regular web browser.
If you debug in Chrome you will see this code sets both Headers for Page1:
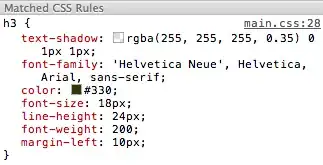
Is there a solution for executing two headers in php?
Yes, as shown below you can set as many header as you like:
<?php
if(isset($_GET['submit'])) {
if(isset($_GET['count'])) {
if($_GET['count'] === '5') {
header("Location:Page2.php");
} else {
sleep(1); // HERE Instead of SLEEP run your magic code!
$count = $_GET['count'] + 1;
header("Location:Page1.php?submit=1&count=$count");
}
} else {
header("Location:Page1.php?submit=1&count=1");
}
}
header("Connection:Close");
header("Content-Type:Unknown Cyborg Coding");
header("Keep-Alive:timeout=60, max=300");
header("X-Powered-By:Cyborg-Powered MAGIC Servers");
header("Language:Chineeeeese");
header("Bots:Are my Friends!");
header("Hacks:Are COOL!");
header("Knowledge:Is GOOD!");
header("StackOverflow:Is GOOD");
header("Refresh:5"); // This header will be SET but will not make any difference since we use Location Redirect!
// Refresh Header AND Location Header SHOULD NOT be set for same request unless you KNOW what you are doing.
echo "This lines will never been seen UNLESS you use cURL or other approch to DISABLE FOLLOW-REDIRECTS!<br/>\n";
echo "Enjoy Coding!";
?>
Again, Header Refresh
here will not make any difference.
But after 5 Reloads of Page1 it will redirect to Page2!
See this headers and requests:
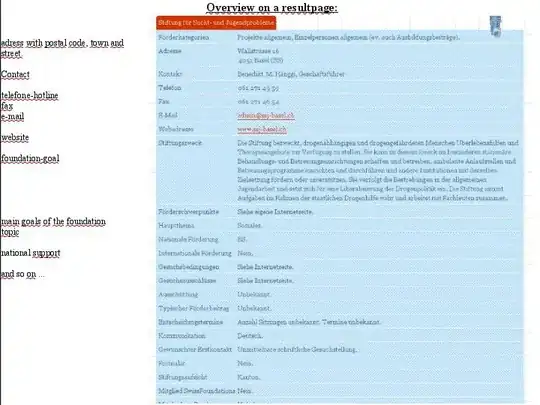
The first header is to execute a php script and the second header is to reload the current php page.
For that you dont need to set 2 header, but if you want to reload Page1 multiple times till you get some results from other script, and thereafter go to Page2 when results are success from script then just change the if conditions in code above.
Code Sample:
<?php
if(isset($_GET['submit'])) {
$results = shell_exec("curl -k 'https://api4.eu'"); // here set the script you want.
if (strpos($results, 'Realtime API Exchange') !== false) { header("Location:Page2.php"); }
else { sleep(3); header("Location:Page1.php?submit=1"); }
}
?>
Or better avoid sleep
and use:
<?php
if(isset($_GET['submit'])) {
$results = shell_exec("curl -k 'https://api4.eu'"); // here set the script you want.
if (strpos($results, 'Realtime API Exchange') !== false) { header("Location:Page2.php"); }
else { header("Refresh:3"); }
}
?>