in your code the
float ld = length(p - pos);
Is computing your distance from light uniformly in all directions (euclidean distance). If you want different shading change the equation...
For example you can compute minimal perpendicular distance to a polygon shaped light like this:
Vertex:
// Vertex
#version 420 core
layout(location=0) in vec2 pos; // glVertex2f <-1,+1>
layout(location=8) in vec2 txr; // glTexCoord2f Unit0 <0,1>
out smooth vec2 t1; // fragment position <0,1>
void main()
{
t1=txr;
gl_Position=vec4(pos,0.0,1.0);
}
Fragment:
// Fragment
#version 420 core
uniform sampler2D txrmap; // texture unit
uniform vec2 t0; // mouse position <0,1>
in smooth vec2 t1; // fragment position <0,1>
out vec4 col;
// light shape
const int n=3;
const float ldepth=0.25; // distance to full disipation of light
const vec2 lpolygon[n]= // vertexes CCW
{
vec2(-0.05,-0.05),
vec2(+0.05,-0.05),
vec2( 0.00,+0.05),
};
void main()
{
int i;
float l;
vec2 p0,p1,p,n01;
// compute perpendicular distance to edges of polygon
for (p1=vec2(lpolygon[n-1]),l=0.0,i=0;i<n;i++)
{
p0=p1; p1=lpolygon[i]; // p0,p1 = edge of polygon
p=p1-p0; // edge direction
n01=normalize(vec2(+p.y,-p.x)); // edge normal CCW
// n01=normalize(vec2(-p.y,+p.x)); // edge normal CW
l=max(dot(n01,t1-t0-p0),l);
}
// convert to light strength
l = max(ldepth-l,0.0)/ldepth;
l=l*l*l;
// render
// col=l*texture2D(txrmap,t1);
col = l*vec4(1.0,1.0,1.0,0.0);
}
I used similar code How to implement 2D raycasting light effect in GLSL as a start point hence the slightly different names of variables.
The idea is to compute perpendicular distance of fragment to all the edges of your light shape and pick the biggest one as the others are facing wrong side.
The lpolygon[n]
is the shape of light relative to light position t0
and the t1
is fragment position. It must be in CCW winding otherwise you would need to
negate the normal computation (mine view is flipped so it might look its CW but its not). I used range <0,1>
as you can use that as texture coordinate directly...
Here screenshot:
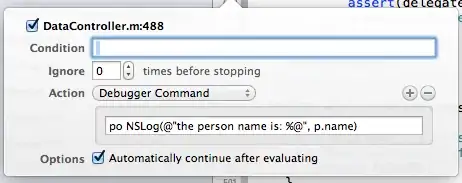
Here some explanations:
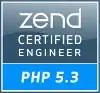
For analytical shape you need to use analytical distance computation ...