I have started learning CSS Grid today and I have a doubt.
When using gap
property the second row is displaying the gaps perfectly but first row's gap are filled with the first column background color. I don't understand why.
div {
height: 50px;
text-align: center;
font-weight: 600;
}
div:nth-child(1) {
background-color: blueviolet;
}
div:nth-child(2) {
background-color: coral;
}
div:nth-child(3) {
background-color: darkgoldenrod;
}
div:nth-child(4) {
background-color: darksalmon;
}
div:nth-child(5) {
background-color: deeppink;
}
div:nth-child(6) {
background-color: gold;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Flexbox</title>
<link rel="stylesheet" href="./index.css" />
<style>
.container {
display: grid;
grid-template-columns: 100px auto 100px;
grid-template-rows: 50px 50px;
gap: 3px;
}
</style>
</head>
<body>
<div class="container">
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
<div>5</div>
<div>6</div>
</div>
</body>
</html>
OUTPUT :
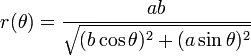