May be this will solve your problem. If Not, Please explain your scenario more detail
Before Going to Actual Implementation Refer the links, How to Loop through the set of records and Cursor
Sample Data for Inventory Table:
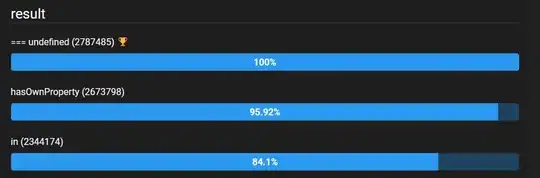
Query For FIFO Basis
Declare @ReqOrderQty as int;
Set @ReqOrderQty=45; // Requested Qty to Update the Records
Declare
@ItemNo Varchar(10),
@MFGCode Varchar(10),
@StockQty int,
@OrderQty int;
Declare @Query Varchar(500);
Declare Records Cursor
for Select ItemNo,MFGCode,StockQty,OrderQty from Inventory where ItemNo='1' and OrderQty <> StockQty order by MFGCode asc
OPEN Records
FETCH NEXT FROM Records INTO
@ItemNo,
@MFGCode,
@StockQty,
@OrderQty;
WHILE @@FETCH_STATUS = 0
BEGIN
IF @ReqOrderQty > @StockQty
BEGIN
Set @ReqOrderQty = @ReqOrderQty - @StockQty;
Set @Query='Update Inventory set OrderQty=' +CAST(@StockQty as varchar(100))+' where ItemNo='''+@ItemNo +'''and MFGCode='''+@MFGCode+''''
END
Else
BEGIN
Set @ReqOrderQty = @ReqOrderQty % @StockQty;
Set @Query='Update Inventory set OrderQty=' +CAST(@ReqOrderQty as varchar(100))+' where ItemNo='''+@ItemNo +'''and MFGCode='''+@MFGCode+''''
END
PRINT @Query
Exec (@Query)
FETCH NEXT FROM Records INTO
@ItemNo,
@MFGCode,
@StockQty,
@OrderQty;
END;
CLOSE Records;
DEALLOCATE Records;
Output
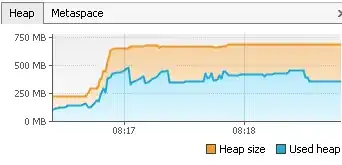
String Split
create table #Temp(value varchar(10))
Declare @ReqOrderQty Varchar(200)
Set @ReqOrderQty = '200,40,10,100,150';
INSERT INTO #Temp SELECT * FROM STRING_SPLIT ( @ReqOrderQty , ',' )
// Perform the Cursor Operation as mentioned above
Drop Table #Temp
Accept the Answer, If it helps you