Not very sure if you mean the probability density function, which is:
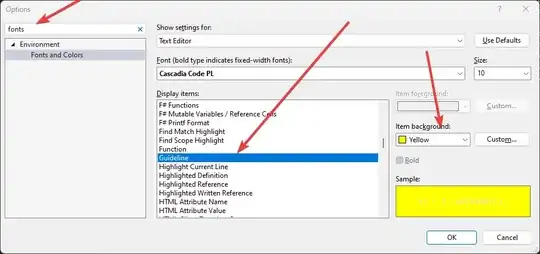
given a certain mean and standard deviation. In python you can use the stats.norm.fit
to get the probability, for example, we have some data where we fit a normal distribution:
from scipy import stats
import seaborn as sns
import numpy as np
import matplotlib.pyplot as plt
data = stats.norm.rvs(10,2,1000)
x = np.linspace(min(data),max(data),1000)
mu, var = stats.norm.fit(data)
p = stats.norm.pdf(x, mu, std)
Now we have estimated the mean and standard deviation, we use the pdf to estimate probability at for example 12.5:
xval = 12.5
p_at_x = stats.norm.pdf(xval,mu,std)
We can plot to see if it is what you want:
fig, ax = plt.subplots(1,1)
sns.distplot(data,bins=50,ax=ax)
plt.plot(x,p)
ax.hlines(p_at_x,0,xval,linestyle ="dotted")
ax.vlines(xval,0,p_at_x,linestyle ="dotted")
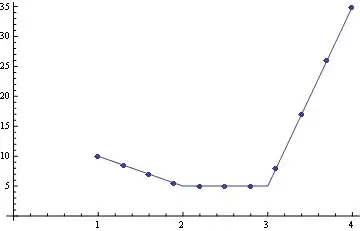