Here you have to keep in mind the internationalisation.
Even if you don't have localised texts now, you may have to support it in the future. So, keeping that in mind, you won't need simply to "split" the string, but to take a specific text from somewhere (i.e. translate it according to the culture).
You can achieve it with the help of some attributes, extension methods and some clever binding.
Let's say that you want to have a picker with 2 options - what is the property type. The PropertyType
is an enum, that looks like this:
public enum PropertyType
{
House,
Apartment
}
Since the built-in Description
attribute can't translate texts for us, we can use a custom attribute to assign a specific text to an enum type, like this:
public enum PropertyType
{
[LocalizedDescription(nameof(R.SingleFamilyHouse))]
House,
[LocalizedDescription(nameof(R.ApartmentBuilding))]
Apartment
}
The attribute code looks like this:
public class LocalizedDescriptionAttribute : DescriptionAttribute
{
private readonly ResourceManager resourceManager;
private readonly string resourceKey;
public LocalizedDescriptionAttribute(string resourceKey, Type resourceType = null)
{
this.resourceKey = resourceKey;
if (resourceType == null)
{
resourceType = typeof(R);
}
resourceManager = new ResourceManager(resourceType);
}
public override string Description
{
get
{
string description = resourceManager.GetString(resourceKey);
return string.IsNullOrWhiteSpace(description) ? $"[[{resourceKey}]]" : description;
}
}
}
R
is my resx file. I have created a Resources
folder and inside it I have 2 resx files - R.resx
(for English strings) & R.de.resx
(for German translation). If you don't want to have internationalisation now, you can change the implementation to get your strings from another place. But it is considered a good practice to always use a resx file, even if you only have 1 language. You never now what tomorrow may bring.
Here is my structure:
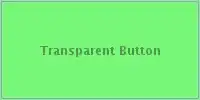
The idea behind LocalizedDescriptionAttribute
class is that the built-in Description
attribute isn't very useful for our case. So we'll have to take the resource key that we have provided it, translates and to override the Description
attribute, which later we will reference.
Now we need to obtain the localised description text with this helper method:
public static class EnumExtensions
{
public static string GetLocalizedDescriptionFromEnumValue(this Enum value)
{
return !(value.GetType()
.GetField(value.ToString())
.GetCustomAttributes(typeof(LocalizedDescriptionAttribute), false)
.SingleOrDefault() is LocalizedDescriptionAttribute attribute) ? value.ToString() : attribute.Description;
}
}
Now, when we create the bindings for the Picker, we won't just use a simple Enum, but a specific PropertyTypeViewModel
, which will have 2 properties - the Enum itself and a Name that will be displayed.
public class PropertyTypeViewModel : BaseViewModel
{
private string name;
public string Name
{
get => name;
set => SetValue(ref name, value);
}
private PropertyType type;
public PropertyType Type
{
get => type;
set => SetValue(ref type, value);
}
public PropertyTypeViewModel()
{
}
public PropertyTypeViewModel(PropertyType type)
: this()
{
Type = type;
Name = type.GetLocalizedDescriptionFromEnumValue();
}
}
The important line is the last one - Name = type.GetLocalizedDescriptionFromEnumValue();
The final thing that is left is to set your Picker's ItemsSource
to your collection of PropertyTypeViewModels and ItemDisplayBinding
to be pointing to the Name
property - ItemDisplayBinding="{Binding Name}"
That's it - now you have a Picker with dynamic localised strings.