So, I've tried a lot of different solutions and came up with this, which worked for my case in Opera, Safari (mobile), firefox, chrome and edge.
My Solution
To get a simliar look, the viewbox will be changed based on the used browser. Therefore, the viewbox values will be divided by "2" (rule of thumb) for safari and edge.
I am not sure, if this is a good solution but I hope someone can work with it or could improve it.
I extended the example with a rectangle and a polygon star. In addition, I added a borders to see the rendered boxes.
var browser = (function (agent) {
switch (true) {
case agent.indexOf("edge") > -1: return "edge";
case agent.indexOf("edg") > -1: return "chromium based edge (dev or canary)";
case agent.indexOf("opr") > -1 && !!window.opr: return "opera";
case agent.indexOf("chrome") > -1 && !!window.chrome: return "chrome";
case agent.indexOf("trident") > -1: return "ie";
case agent.indexOf("firefox") > -1: return "firefox";
case agent.indexOf("safari") > -1: return "safari";
default: return "other";
}
})(window.navigator.userAgent.toLowerCase());
console.log(browser);
function resetSVGIconViewBox(elemId){
console.log(elemId);
if(jQuery("#" + elemId).length == 0 || !jQuery("#" + elemId).attr('viewBox'))
{
return;
}
console.log(jQuery("#" + elemId)[0].getAttribute('viewBox'));
let newViewBoxValues = jQuery("#" + elemId)[0].getAttribute('viewBox').split(" ").map(x => x / 2);
jQuery("#" + elemId)[0].setAttribute('viewBox', newViewBoxValues.join(' '));
console.log(newViewBoxValues.join(' '));
}
if(browser == "edge" || browser == "safari")
{
resetSVGIconViewBox("icon-search");
resetSVGIconViewBox("test-bug");
resetSVGIconViewBox("poly-id");
resetSVGIconViewBox("rect-id");
}
else
{
console.log("no viewbox changes")
}
/* given classes of third party libs */
svg:not(:root) {
overflow: hidden;
}
.icon-search {
width: 1em;
font-size: 14px;
}
.icon {
display: inline-block;
height: 1em;
stroke-width: 0;
border: 1px red solid;
}
/* given classes of third party libs */
/* only for testing*/
p{
border: 1px red solid;
}
i{
font-style: normal;
}
/* only for testing*/
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.2.3/jquery.min.js"></script>
<svg style="display:none;" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink">
<defs>
<symbol id="rect-id" viewBox="0 0 100 100" width="30px" height="30px">
<rect width="100%" height="100%"/>
</symbol>
<symbol id="poly-id" viewBox="20 35 320 320" width="30px" height="30px">
<polygon points="100,10 40,198 190,78 10,78 160,198"/>
</symbol>
<symbol id="icon-search" viewBox="0 0 56 60" width="30px" height="30px"><path id="font-awesome-svg-search" d="M18 13c0-3.859-3.141-7-7-7s-7 3.141-7 7 3.141 7 7 7 7-3.141 7-7zM26 26c0 1.094-0.906 2-2 2-0.531 0-1.047-0.219-1.406-0.594l-5.359-5.344c-1.828 1.266-4.016 1.937-6.234 1.937-6.078 0-11-4.922-11-11s4.922-11 11-11 11 4.922 11 11c0 2.219-0.672 4.406-1.937 6.234l5.359 5.359c0.359 0.359 0.578 0.875 0.578 1.406z"></path></symbol>
</defs>
</svg>
<p>
<i>
Test
</i>
<svg class="icon icon-search">
<use xlink:href="#rect-id">
</use>
</svg>
<svg class="icon icon-search">
<use xlink:href="#poly-id">
</use>
</svg>
<svg class="icon icon-search">
<use xlink:href="#icon-search">
</use>
</svg>
</p>
Testing
I've tested safari mobile on my iPhone 7 with jsfiddle. By enabling mobile toolbar in the chrome developer mode for iPhone, the code will return "safari" but the chrome browser is still using the internal chrome engine.
For me, it is not possible to run the snippet on the mobile stackoverflow page. If I change to the "full site", the result is as shown in jfiddle.
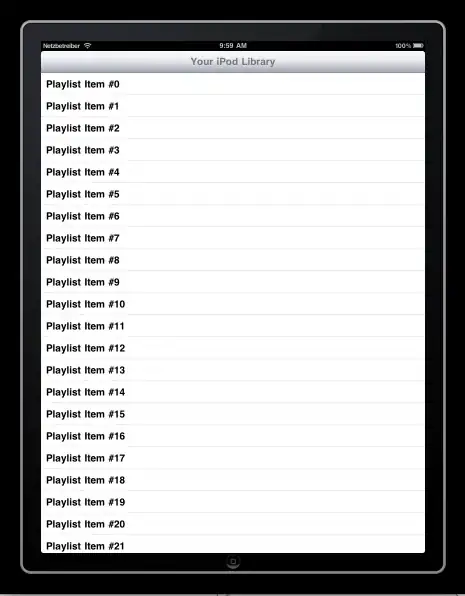
The solution could be a mess but it works for me as a workaround.