You can use Reflection
to achieve your requirement as @Józef Podlecki said.
You can also refer to this.
Here is a generic method to achieve it:
public class PerInfo
{
[StringLength(64, MinimumLength = 8, ErrorMessage = "{0} must be between {2} to {1} characters")]
public string Password { get; set; }
}
As the response format you provided, you can create a error model:
public class CusError
{
public long errorCode { get; set; }
public string message { get; set; }
public string[] args { get; set; }
}
Code:
[HttpPost]
public IActionResult Validate(PerInfo perInfo)
{
if (!ModelState.IsValid)
{
var error = GenerateValidationModel<PerInfo>();
return BadRequest(error);
}
return Ok();
}
private CusError GenerateValidationModel<T>()
{
var error = new CusError();
foreach (var prop in typeof(T).GetProperties())
{
object[] attrs = prop.GetCustomAttributes(true);
if (attrs == null || attrs.Length == 0)
continue;
foreach (Attribute attr in attrs)
{
if (attr is StringLengthAttribute)
{
error.errorCode = 1234;
error.message = string.Format((attr as StringLengthAttribute).ErrorMessage, prop.Name, (attr as StringLengthAttribute).MaximumLength.ToString(), (attr as StringLengthAttribute).MinimumLength.ToString());
error.args = new string[] { (attr as StringLengthAttribute).MinimumLength.ToString(), (attr as StringLengthAttribute).MaximumLength.ToString() };
}
}
}
return error;
}
Here is the test result by postman:
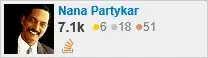