Not sure what you're looking for exactly, but best software practices be damned, here you go (assuming solution is called "stackoverflow61918396"):
Student.cs
using System.Collections.Generic;
namespace stackoverflow61918396
{
class Student
{
public string Id { get; set; }
public string Name { get; set; }
public List<string> SubjectsEnrolledIn { get; set; }
public Student()
{
SubjectsEnrolledIn = new List<string>();
}
}
}
Subject.cs
using System.Collections.Generic;
namespace stackoverflow61918396
{
class Subject
{
public string Id { get; set; }
public int ClassNumber { get; set; }
public string Name { get; set; }
public List<string> StudentsInSubject { get; set; }
public Subject()
{
StudentsInSubject = new List<string>();
}
}
}
Manager.cs
using System;
using System.Collections.Generic;
using System.Linq;
namespace stackoverflow61918396
{
class Manager
{
public static List<Student> Students { get; set; }
public static List<Subject> Subjects { get; set; }
public Manager()
{
Students = new List<Student>();
Subjects = new List<Subject>();
// Seed some students
var ben = new Student() { Id = Guid.NewGuid().ToString(), Name = "Ben" };
var andrew = new Student() { Id = Guid.NewGuid().ToString(), Name = "Andrew" };
var amanda = new Student() { Id = Guid.NewGuid().ToString(), Name = "Amanda" };
var greg = new Student() { Id = Guid.NewGuid().ToString(), Name = "Greg" };
var peter = new Student() { Id = Guid.NewGuid().ToString(), Name = "Peter" };
var jessica = new Student() { Id = Guid.NewGuid().ToString(), Name = "Jessica" };
var james = new Student() { Id = Guid.NewGuid().ToString(), Name = "James" };
var doug = new Student() { Id = Guid.NewGuid().ToString(), Name = "Doug" };
var cory = new Student() { Id = Guid.NewGuid().ToString(), Name = "Cory" };
var laura = new Student() { Id = Guid.NewGuid().ToString(), Name = "Laura" };
Students.Add(ben);
Students.Add(andrew);
Students.Add(amanda);
Students.Add(greg);
Students.Add(peter);
Students.Add(jessica);
Students.Add(james);
Students.Add(doug);
Students.Add(cory);
Students.Add(laura);
// Seed a couple sections of math and science
var math2 = new Subject() { Id = Guid.NewGuid().ToString(), Name = "Math", ClassNumber = 2 };
var math4 = new Subject() { Id = Guid.NewGuid().ToString(), Name = "Math", ClassNumber = 4 };
var science5 = new Subject() { Id = Guid.NewGuid().ToString(), Name = "Science", ClassNumber = 5 };
var science3 = new Subject() { Id = Guid.NewGuid().ToString(), Name = "Science", ClassNumber = 3 };
Subjects.Add(math2);
Subjects.Add(math4);
Subjects.Add(science5);
Subjects.Add(science3);
// Seed some enrollments
Enroll(ben.Id, math2.Id);
Enroll(andrew.Id, math2.Id);
Enroll(andrew.Id, science5.Id);
Enroll(amanda.Id, math2.Id);
Enroll(peter.Id, math2.Id);
Enroll(jessica.Id, math4.Id);
Enroll(james.Id, math2.Id);
Enroll(doug.Id, math4.Id);
Enroll(doug.Id, science5.Id);
}
public Student AddStudent(string name)
{
var student = new Student() { Id = Guid.NewGuid().ToString(), Name = name };
Students.Add(student);
return student;
}
public Subject AddSubject(string name, int classNumber)
{
var subject = new Subject() { Id = Guid.NewGuid().ToString(), Name = name, ClassNumber = classNumber };
Subjects.Add(subject);
return subject;
}
public bool DeleteStudent(string id)
{
try
{
var student = Students.Where(s => s.Id == id).FirstOrDefault();
if (student == null) return false;
Students.Remove(student);
return true;
}
catch (Exception)
{
return false;
}
}
public bool DeleteSubject(string id)
{
try
{
var subject = Subjects.Where(s => s.Id == id).FirstOrDefault();
if (subject == null) return false;
Subjects.Remove(subject);
return true;
}
catch (Exception)
{
return false;
}
}
public bool Disenroll(string studentId, string subjectId)
{
var student = Students.Where(s => s.Id == studentId).First();
var subject = Subjects.Where(s => s.Id == subjectId).First();
try
{
student.SubjectsEnrolledIn.Remove(subjectId);
subject.StudentsInSubject.Remove(studentId);
}
catch (Exception)
{
return false;
}
return true;
}
public Student EditStudent(string id, string name)
{
var student = Students.Where(s => s.Id == id).FirstOrDefault();
if (student == null) return null;
student.Name = name;
return student;
}
public Subject EditSubject(string id, string name, int classNumber)
{
var subject = Subjects.Where(s => s.Id == id).FirstOrDefault();
if (subject == null) return null;
subject.Name = name;
subject.ClassNumber = classNumber;
return subject;
}
public bool Enroll(string studentId, string subjectId)
{
var student = Students.Where(s => s.Id == studentId).First();
var subject = Subjects.Where(s => s.Id == subjectId).First();
try
{
student.SubjectsEnrolledIn.Add(subjectId);
subject.StudentsInSubject.Add(studentId);
}
catch (Exception)
{
return false;
}
return true;
}
public Student GetStudent(string id)
{
return Students.Where(s => s.Id == id).FirstOrDefault();
}
public Subject GetSubject(string id)
{
return Subjects.Where(s => s.Id == id).FirstOrDefault();
}
public IEnumerable<IGrouping<string, Tuple<string, int, string>>> GetSubjectAllStudents(string id)
{
var tupleList = new List<Tuple<string, int, string>>();
var subjectName = Subjects.Where(s => s.Id == id).FirstOrDefault()?.Name;
var subjectList = Subjects.Where(s => s.Name == subjectName).ToList();
if (subjectName == null) return null;
foreach (Subject subject in subjectList)
{
foreach (Student student in Students)
{
if (subject.StudentsInSubject.Contains(student.Id))
{
tupleList.Add(new Tuple<string, int, string>(student.Name, subject.ClassNumber, "yes"));
}
else
{
tupleList.Add(new Tuple<string, int, string>(student.Name, subject.ClassNumber, "no"));
}
}
}
var sortedTupleList = tupleList.GroupBy(x => x.Item1);
return sortedTupleList;
}
public List<Subject> GetSubjectAlternatives(string id)
{
var subjectName = Subjects.Where(s => s.Id == id).FirstOrDefault()?.Name;
if (subjectName == null) return null;
return Subjects.Where(s => s.Name == subjectName).ToList();
}
public List<Student> ListStudents()
{
return Students;
}
public List<Subject> ListSubjects()
{
return Subjects;
}
}
}
Program.cs
using System;
using System.Collections.Generic;
namespace stackoverflow61918396
{
class Program
{
static bool Again { get; set; }
static Manager Manager { get; set; }
static void Main()
{
Again = true;
Manager = new Manager();
do
{
Console.WriteLine("Enter a command (type 'help' for options):");
var selection = Console.ReadLine();
Console.WriteLine("\n");
CommandHandler(selection);
} while (Again);
}
static void CommandHandler(string command)
{
switch (command)
{
case var s when s.Trim().ToLower() == "help":
Console.WriteLine("Commands:");
Console.WriteLine("'quit' - exits the program");
Console.WriteLine("'students' - lists all students");
Console.WriteLine("'subjects' - lists all subjects");
Console.WriteLine("'student [id]' - shows this student's record");
Console.WriteLine("'subject [id]' - shows this subject's record");
Console.WriteLine("'all students by subject [id]' - lists all students in this subject or not");
Console.WriteLine("'alternatives for subject [id]' - lists all class numbers of a subject");
Console.WriteLine("'new student [name]' - adds a new student");
Console.WriteLine("'new subject [name] [class number]' - adds a new subject");
Console.WriteLine("'edit student [id] [name]' - edits an existing student");
Console.WriteLine("'edit subject [id] [name] [class number]' - edits an existing subject");
Console.WriteLine("'delete student [id]' - deletes an existing student");
Console.WriteLine("'delete subject [id]' - deletes an existing subject");
Console.WriteLine("'enroll [student id] [subject id]' - enrolls a student into a subject");
Console.WriteLine("'disenroll [student id] [subject id]' - disenrolls a student from a subject");
Console.WriteLine("\n");
break;
case var s when s.Trim().ToLower() == "quit":
Again = false;
break;
case var s when s.Trim().ToLower() == "students":
try
{
List<Student> studentList = Manager.ListStudents();
if (studentList == null) throw new Exception();
Console.WriteLine("Students:");
foreach (Student student in studentList)
{
Console.WriteLine("Id: " + student.Id + ", Name: " + student.Name);
}
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower() == "subjects":
try
{
List<Subject> subjectList = Manager.ListSubjects();
if (subjectList == null) throw new Exception();
Console.WriteLine("Subjects:");
foreach (Subject subject in subjectList)
{
Console.WriteLine(
"Id: " + subject.Id +
", Class Number: " + subject.ClassNumber +
", Name: " + subject.Name
);
}
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("student"):
try
{
var studentId = s.Split(' ')[1];
var student = Manager.GetStudent(studentId);
if (student == null) throw new Exception();
Console.WriteLine("Id: " + student.Id + ", Name: " + student.Name);
Console.WriteLine("Subjects Enrolled In:");
foreach (string subjectId in student.SubjectsEnrolledIn)
{
var subject = Manager.GetSubject(subjectId);
Console.WriteLine(
"Id: " + subject.Id +
", Class Number: " + subject.ClassNumber +
", Name: " + subject.Name
);
}
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("subject"):
try
{
var subjectId = s.Split(' ')[1];
var subject = Manager.GetSubject(subjectId);
if (subject == null) throw new Exception();
Console.WriteLine("Id: " + subject.Id + ", Name: " + subject.Name);
Console.WriteLine("Students In Subject:");
foreach (string studentId in subject.StudentsInSubject)
{
var student = Manager.GetStudent(studentId);
Console.WriteLine(
"Id: " + student.Id +
", Name: " + student.Name
);
}
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("all"):
try
{
var subjectId = s.Split(' ')[4];
var subjectName = Manager.GetSubject(subjectId)?.Name;
var subjectWithAll = Manager.GetSubjectAllStudents(subjectId);
if (subjectWithAll == null) throw new Exception();
Console.WriteLine("All students with respect to " + (subjectName ?? subjectId) + ":");
foreach (var group in subjectWithAll)
{
foreach (var item in group)
{
Console.WriteLine(
"\tName: " + item.Item1 +
", \t\tClass Number: " + item.Item2 +
", \t\tEnrolled: " + item.Item3
);
}
}
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("alternatives"):
try
{
var subjectId = s.Split(' ')[3];
List<Subject> subjectAlternatives = Manager.GetSubjectAlternatives(subjectId);
if (subjectAlternatives == null) throw new Exception();
Console.WriteLine("Alternatives for " + subjectAlternatives[0].Name + ":");
foreach (Subject subject in subjectAlternatives)
{
Console.WriteLine("Id: " + subject.Id + ", Class Number: " + subject.ClassNumber);
}
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("new student"):
try
{
var name = s.Split(' ')[2];
var student = Manager.AddStudent(name);
if (student == null) throw new Exception();
Console.WriteLine("Student " + student.Id + " created");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("new subject"):
try
{
var name = s.Split(' ')[2];
var classNumber = s.Split(' ')[3];
var subject = Manager.AddSubject(name, int.Parse(classNumber));
if (subject == null) throw new Exception();
Console.WriteLine("Subject " + subject.Id + " created");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("edit student"):
try
{
var studentId = s.Split(' ')[2];
var studentName = s.Split(' ')[3];
var student = Manager.EditStudent(studentId, studentName);
if (student == null) throw new Exception();
Console.WriteLine("Student " + student.Id + " was edited");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("edit subject"):
try
{
var subjectId = s.Split(' ')[2];
var subjectName = s.Split(' ')[3];
var subjectClassNumber = s.Split(' ')[4];
var subject = Manager.EditSubject(subjectId, subjectName, int.Parse(subjectClassNumber));
if (subject == null) throw new Exception();
Console.WriteLine("Subect " + subject.Id + " was edited");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("delete student"):
try
{
var studentId = s.Split(' ')[2];
var deleted = Manager.DeleteStudent(studentId);
Console.WriteLine("Student was " + (deleted ? "" : "not") + " deleted");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("delete subject"):
try
{
var subjectId = s.Split(' ')[2];
var deleted = Manager.DeleteSubject(subjectId);
Console.WriteLine("Subject was " + (deleted ? "" : "not") + " deleted");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("enroll"):
try
{
var studentId = s.Split(' ')[1];
var subjectId = s.Split(' ')[2];
var enrolled = Manager.Enroll(studentId, subjectId);
Console.WriteLine("Student was " + (enrolled ? "" : "not") + " enrolled");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
case var s when s.Trim().ToLower().StartsWith("disenroll"):
try
{
var studentId = s.Split(' ')[1];
var subjectId = s.Split(' ')[2];
var disenrolled = Manager.Disenroll(studentId, subjectId);
Console.WriteLine("Student was " + (disenrolled ? "" : "not") + " disenrolled");
Console.WriteLine("\n");
}
catch (Exception)
{
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
}
break;
default:
Console.WriteLine("Unrecognized command. Please try again.");
Console.WriteLine("\n");
break;
}
}
}
}
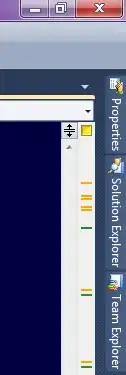