You can remove each legend for the first three axes, and then use plt.figlegend()
which is a legend for the entire figure. You might have to adjust the bbox_to_anchor()
arguments based on what is in your legend. (Please ignore the details of my plots which were used solely for illustrative purposes.)
import seaborn as sns
df = sns.load_dataset('flights')
fig, ax = plt.subplots(2,2)
ax1 = sns.lineplot(x=df['year'],y=df['passengers'],
color='b',dashes=False,label='ax 1 line',ax=ax[0,0])
ax2 = sns.lineplot(x=df['year'],y=df['passengers'],
color='r',dashes=False,label='ax 2 line',ax=ax[0,1])
ax3 = sns.lineplot(x=df['year'],y=df['passengers'],
color='g',dashes=False,label='ax 3 line',ax=ax[1,0])
ax1.get_legend().remove()
ax2.get_legend().remove()
ax3.get_legend().remove()
plt.figlegend(loc='lower right',bbox_to_anchor=(0.85,0.25))
plt.show()
Result:
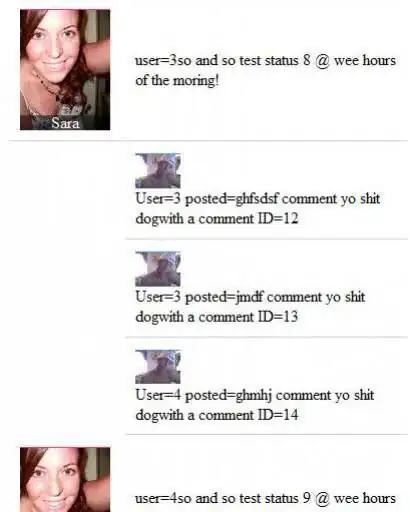