Not quite sure why the UnityEngine
namespace should not not exist in any Unity made project - on any target platform.
It is the main engine core so why do you think it should not be there?
Pre-Processor that covers any Unity version (since 5.3.4)
Anyway from using the general #if
pre-processors (See Unity Manual - Platform dependent compilation) starting from Unity version 5.3.4
you can check whether you are in any Unity version by simply using
#if UNITY_5_3_OR_NEWER
// Use UnityEngine
#else
// Use something else
#endif
which as the name says covers all Unity versions above 5.3.4
and any project/app built with it regardless of the target device.
Use a global define
Also instead of your own
#define UNITY
in each and every script you can go to the Player Settings (Edit → Project Settings → Player) and add your own global custom define in the Unity Editor (and a build of course) for all scripts:
Open the Other Settings
panel of the Player Settings
and navigate to the Scripting Define Symbols
text box.
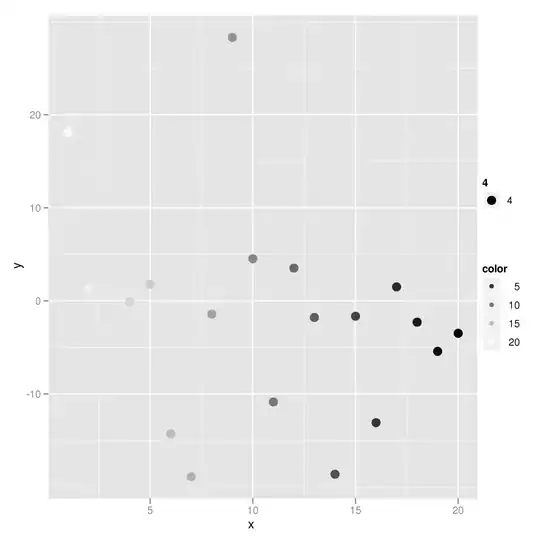
Here you can add a ;
-separated list of your own global defines. There you could also simply enter UNITY
and then use
#if UNITY
// Use Unity stuff
#else
// Use other stuff
#endif
Add global define automatically via Editor script
And now the cool thing: You can add such a global define also via editor script!
Therefore e.g. simply add a script in your library within a folder called Editor
:
#if !YOURDEFINE
// We only need this class once if the global define is not added yet
// The above YOURDEFINE has to exactly match the DEFINE declared below
// Once it is already defined in the PlayerSettings it stays defined permanently
// so we don't need to run this class on every project load
using UnityEngine;
using UnityEditor;
internal class StartUp
{
/// <summary>
/// The define to be added to the global defines list.
/// <para>This needs to match exactly the #if pre-processor
/// on top of this file!</para>
/// </summary>
private const DEFINE = "YOURDEFINE";
/// <summary>
/// automatically executed once the project is loaded and after
/// every re-compile in the UnityEditor
/// </summary>
[InitializeOnLoadMethod]
private static void Initialize()
{
// Get current defines from PlayerSettings
var defines = PlayerSettings.GetScriptingDefineSymbolsForGroup(EditorUserBuildSettings.selectedBuildTargetGroup);
// Split into list at ';'
var defineList = defines.Split(';').ToList();
// Check if already contains your define
// Actually this should never happen since if this is the case
// The entire class should already be stripped of due to the
// pre-processor on top of this file
// Therefore if this happens something is wrong
if (defineList.Contains(Constants.Define))
{
Debug.LogError("Huh?! This should not happen! Please check the DEFINE const and the pro-processor on top of StartUp.cs!");
return;
}
// if not simply append it
// Unity doesn't care about a leading ;
// if this is the first entry it is removed automatically
defines += $";{DEFINE}";
// This writes back the new defines and evtl. causes a re-compile
// be careful with that though because if you mess it up
// you might end up in a permanent re-compile ;)
PlayerSettings.SetScriptingDefineSymbolsForGroup(EditorUserBuildSettings.selectedBuildTargetGroup, defines);
}
}
#endif