First of all, as other answer suggests, currently Intent.ACTION_SEND_MULTIPLE
is the way to send multiple files.
But, not having a feature in built-in blocks of Sketchware is not really the exact limitation of the app, as it provides following block which is able to do anything you want in the android way.
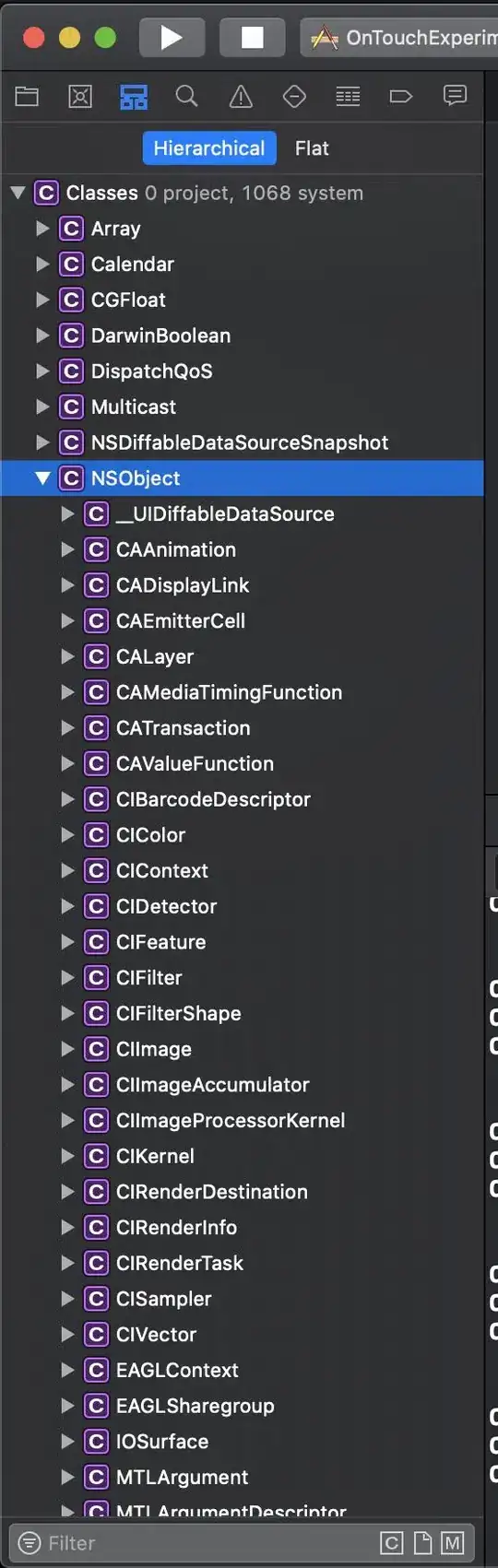
And you have already used this element for adding some custom code. So, for solving your problem, the block will be like this:
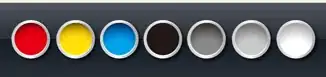
And here is the details of some of the custom code blocks I've added:
mail.setAction(Intent.ACTION_SEND_MULTIPLE):
This custom code has been added by removing default Intent > setAction block. And the action name says it all, this allows sending multiple files through intent.
ArrayList<Uri> uris = new ArrayList<Uri>():
This declares a new ArrayList to store the list of all Uri to be sent through intent.
uris.add(Uri.fromFile(new java.io.File(Environment.getExternalStorageDirectory() + "/Documents/filename1.pdf"))):
This line adds the provided uri to the ArrayList named uris. Call this block as many times as you want to add multiple number of files uri to the list.
mail.putParcelableArrayListExtra(Intent.EXTRA_STREAM, uris):
This binds the uris to the EXTRA_STREAM of the intent.
Edit:
Starting from Android 7.0 and up, there is some policy change for security purpose. That's why this extra code is added. The block image above is updated with this code already:
StrictMode.VmPolicy.Builder builder = new StrictMode.VmPolicy.Builder();
StrictMode.setVmPolicy(builder.build());
Though it is advised to use android.support.v4.content.FileProvider
to solve this type of issue, but for less support in Sketchware platform, at this point it is better to use above method.
You can read this for more clarification of above fix.