The assignments that your are doing is wrong. Basically a pointer points to a block of memory. from your code I can understand that array1 = array2;
and *array1 = &array2;
is wrong.
Syntax in C is something like this data-type* pointer-variable = (data-type*)malloc(no. of bytes you want);
See consider you want 10 block of memory of type int
int *p = (int *)malloc(10 * sizeof(int))
sizeof(int)
return 4 bytes.
Now p
points to 10 * 4 = 40 bytes of memory, I multiplied by 4 because int
is usually of 4 bytes and double
is of 8 bytes and so on.
Follow this link to understand C - Data Types
Now regarding changing pointers refer below example and read the comments
int *q = NULL // declare a pointer of same type as the block of memory it is going to point
q = p; //now q and p point same memory of 40 bytes, means value at q[0] is equal to p[0]
When you have an integer pointer and you increment it by p++
it will point to next memory location p[1]
, pointer will be exactly incremented by 4 bytes as int
size is 4 bytes and for double
it will be 8 bytes, for char
it will be 1 byte and so on.
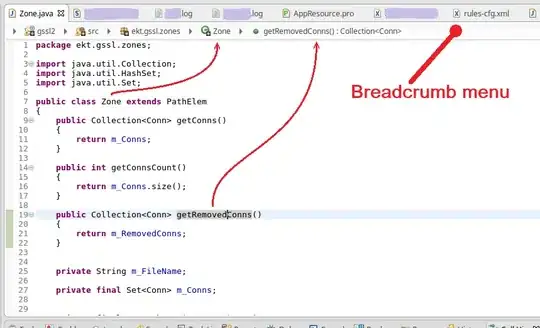
Now if you want to increase the size of dynamically allocated memory you can use realloc
please follow this link to understand more.
Dynamic Memory Allocation in C
int *p = NULL;
// Dynamically allocate memory using malloc()
p = (int*)malloc(no. of bytes, sizeof(int));
// Dynamically re-allocate memory using realloc()
p = realloc(p, (no. of bytes) * sizeof(int));
// Avoid memory leaks
free(p);

Syntax in C++ is something like this data-type* pointer-variable = new data-type[size];
See consider you want 10 block of memory of type int
int *p = new int[10]
Just use new
operator to allocate block of memory and use delete
to free allocated memory to avoid memory leaks.follow this link
new and delete operators in C++ for dynamic memory
Or If you are looking for containers where you don't know how much memory should be allocated the use standard template library vector
, it helps creating dynamic arrays.follow this link
Vector in C++ STL