You can use the below regex to achieve your purpose:
^[\w][\S]{0,8}$
Explanation of the above regex:
^
- Represents the start of the line.
[\w]
- Matches a character from [0-9a-zA-Z_]. If you do not want _
(underscore) then provide the character class manually.[0-9A-Za-z]
[\S]{0,8}
- Matches any non-space character 0 to 8 times.
$
- Represents end of the line.
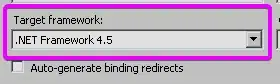
You can find the demo of the above regex here.
Implementation in java:(You can modify the code accordingly to suit your requirements)
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class Main
{
private static final Pattern pattern = Pattern.compile("^[\\w][\\S]{0,8}$", Pattern.MULTILINE);
public static void main(String[] args) {
final String string = "Hello!@+\n"
+ "t123123\n"
+ "H123!#\n"
+ "@adadsa\n"
+ "@3asdasd\n"
+ "%sdf\n"
+ "Helloworld1";
Matcher matcher = pattern.matcher(string);
while(matcher.find())
System.out.println(matcher.group(0));
}
}
You can find the sample run of the above implementation in here.