tl;dr
Generate text representing the date.
LocalDate // Represent a date-only value, without time-of-day and without time zone, using `java.time.LocalDate` class.
.of( 1982 , 1 , 24 ) // Specify a date. Notice the sane numbering of both year and month, unlike the legacy classes `Date`/`Calendar`. Returns a `LocalDate` object.
.format( // Generate text to represent the value of the `LocalDate` object.
DateTimeFormatter
.ofLocalizedDate( FormatStyle.MEDIUM ) // How long or abbreviated do you want the text.
.withLocale( Locale.UK ) // Specify a `Locale` to determine the human language and cultural norms to use in localizing.
) // Returns a `String` object.
24 Jan 1982
Calculate age.
Period
.between(
LocalDate.of( 1982 , 1 , 24 ) ,
LocalDate.now( ZoneId.systemDefault() )
)
.getYears()
Birthday toast.
MonthDay
.now(
ZoneId.systemDefault() // Specify time zone. For any given moment, the date varies around the globe by time zone.
)
.equals(
MonthDay.of( 1 , 24 )
)
java.time
The modern approach uses the java.time classes.
From your DatePicker
, pull the year, month, and day, passing all three to the factory method for LocalDate
.
DatePicker dp = … ;
…
LocalDate localDate = LocalDate.of( dp.getYear() , dp.getMonth() , dp.getDayOfMonth() ) ;
You can define your own formatting pattern when generating text for presentation to the user. But generally better to let java.time automatically localize.
LocalDate localDate = LocalDate.of( 1982 , 1 , 24 ) ;
Locale locale = Locale.UK ;
DateTimeFormatter f = DateTimeFormatter.ofLocalizedDate( FormatStyle.MEDIUM ).withLocale( locale ) ;
String output = localDate.format( f ) ;
See this code run live at IdeOne.com.
24 Jan 1982
To calculate age, look for the LocalDate
related answers on the question: How do I calculate someone's age in Java?.
To see if today is their birthday, use MonthDay
class.
MonthDay birthday = MonthDay.from( localDate ) ;
ZoneId z = ZoneId.of( "America/Montreal" ) ; // The time zone through which we want to see today's date.
MonthDay monthDay = MonthDay.now( z ) ;
if( monthDay.equals( birthday ) ) { … Happy Birthday … }
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?
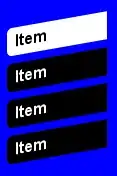