What you want cannot be done for a LOCAL variable like my_variable
.
If that variable is at CLASS level, though, it can be done with REFLECTION.
If the variable is at class level and is PUBLIC, you can cheat and use CallByName:
Public Class Form1
Public counter As Integer = 911
Public my_variable As String = "Hello World!"
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim variable As String = TextBox1.Text
Try
Dim value As String = CallByName(Me, variable, CallType.Get)
MessageBox.Show(variable & " = " & value)
Catch ex As Exception
MessageBox.Show("Variable not found: " & variable)
End Try
End Sub
End Class
Type the name of the variable in TextBox1 and its value will be displayed in the message box....easy peasy.
If you don't want the variables to be public, then it can be accomplished via Reflection, but it doesn't look quite as simple and pretty. Look it up if you want to go that route.
--- EDIT ---
Here's a version that can find public members of a module:
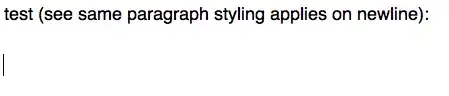
Code:
Imports System.Reflection
Public Class Form2
Public counter As Integer = 911
Public my_variable As String = "Hello World!"
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
lblResults.Text = ""
Dim variable As String = TextBox1.Text
Try
Dim value As String = CallByName(Me, variable, CallType.Get)
lblResults.Text = variable & " = " & value
Catch ex As Exception
End Try
End Sub
Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click
lblResults.Text = ""
Dim moduleName As String = txtModule.Text
Dim moduleField As String = txtModuleMember.Text
Dim myType As Type = Nothing
Dim myModule As [Module] = Nothing
For Each x In Assembly.GetExecutingAssembly().GetModules
For Each y In x.GetTypes
If y.Name.ToUpper = moduleName.ToUpper Then
myType = y
Exit For
End If
Next
If Not IsNothing(myType) Then
Exit For
End If
Next
If Not IsNothing(myType) Then
Dim flags As BindingFlags = BindingFlags.IgnoreCase Or BindingFlags.NonPublic Or BindingFlags.Public Or BindingFlags.Static Or BindingFlags.Instance
Dim fi As FieldInfo = myType.GetField(moduleField, flags)
If Not IsNothing(fi) Then
Dim value As String = fi.GetValue(Nothing)
lblResults.Text = moduleField & " = " & value
End If
End If
End Sub
End Class
Public Module PutThings
Public SomeValue As String = "...success!..."
End Module