To summarize before I go deeper into explanation of what you should do next, let me first describe how WebSockets actually work and where you currently are.
To establish a WebSocket connection, you first need to "talk" to the server using HTTP, as the way WebSockets work is that you send a HTTP request to the server saying "let's talk using WebSockets", at which point server responds with "OK, let's switch protocols" and only at this point it's possible to start sending data using WebSockets. Going further "down the stack", HTTP connections are established over TCP or TLS connections - depending on whether you need things like one- or two-way authentication and data encryption. Therefore you'll need to have TCP or TLS (which in essence really is "extended" TCP) working before you can even get to the HTTP part.
Assuming you've done everything above and have working WebSocket connection established with the server - it actually may be quite tedious for you to exchange chat data using just raw WebSockets and nothing on top of that. Sure, if you plan to exchange just raw chat messages and nothing more, that's fine. However, once additional requirements start piling up - things like: let's send message timestamp, sender name, picture support, file support etc. - it's going to get really annoying to extend really quickly, as in essence you'll have to start developing your own protocol that's sent over WebSockets. The reason is: simple raw WebSockets give you nothing more other than "server sent you X number of bytes and here they are". There's no notion of key-value headers, "body" section of the message or anything similar, just raw data transport mechanism. In addition, depending on what kind of technology stack your server uses (or you're planning to use yourself if you're the developer of the server backend), it may be harder to make it use raw WebSockets rather that something running on top of them. To give you my experience with this - personally I'd recommend something like a STOMP protocol. It's simple enough even to implement its basic mechanisms in about a day or two, but gives you much more flexibility.
To summarize everything above, here's the "big picture" of what's ahead of you:
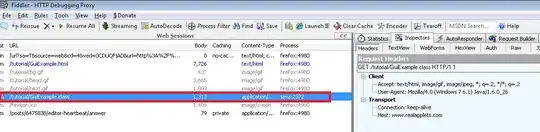
Now (finally) going bottom-up to where you are and what your next steps should be:
You've mentioned that you've successfully connected to the GSM network using some kind of modem. I assume you've only "connected to network" / BTS using AT commands but didn't yet establish data connection - to get to the "I have TCP working" part. Here you can take two paths:
- Use modem's AT commands to open, send and receive TCP data. Some modems have an integrated TCP/IP stack and have AT commands like those mentioned available. Upside: it's faster if your goal is to "just have it done". Downside: some modems don't offer this functionality, commands may sometimes not behave as you expect them to compared to things like usual socket APIs, there may be differences between modems (if you decide to change yours at some point), you don't control any "magic" that happens in the modem's TCP/IP stack and considering errors in this area aren't actually as rare as one could initially think - it may be annoying.
- Use PPP and integrate modem with a TCP/IP stack running on your microcontroller. Here I gave a broader explanation of how to integrate a modem with TCP/IP stack.
After completing either of the steps above, you should have the ability to establish a TCP (or TLS if you decide to go down that path) connection with your server. Once the connection is established in order to get to the WebSocket part, you need to send a HTTP request - therefore you need a HTTP client. Again two possible paths: either get HTTP client written by somebody and that can run on your limited microcontroller resources, or write one yourself - at least capable of sending a very simple HTTP GET request. This isn't as scary as it sounds. To ask server to switch to WebSocket, the request looks something like this:
GET /chat HTTP/1.1
Host: server.example.com
Connection: Upgrade
Upgrade: websocket
Sec-WebSocket-Key: x3JJHMbDL1EzLkh9GBhXDw==
Sec-WebSocket-Protocol: chat, superchat
Sec-WebSocket-Version: 13
Origin: http://example.com
Once server responds, you should have a working WebSocket connection at which point you implement (or get a ready implementation) of WebSocket protocol and possibly STOMP/similar/your own protocol running on top of that.