An initial setting of a flex container is align-content: stretch
. This means that flex lines (i.e., "rows" or "columns") will expand the cross axis of the container equally. And that's what you get when you don't set any heights on the items:
.flex-container {
display: flex;
flex-wrap: wrap;
width: 400px;
height: 200px;
border: 1px solid black;
align-items: stretch;
}
.flex-container> :nth-child(1) {
background-color: darkorchid;
/* height: 60px; */
}
.flex-container > :nth-child(2) {
background-color: darkkhaki;
}
.flex-container > :nth-child(3) {
background-color: dodgerblue;
}
.flex-container > :nth-child(4) {
background-color: darkgoldenrod;
}
.flex-container > :nth-child(5) {
background-color: darkcyan;
}
<div class="flex-container">
<span>I am number 1</span>
<div>I am number 2</div>
<div>I am number 3</div>
<div>I am number 4</div>
<span>I am number 5</span>
</div>
In a container with height: 200px
and align-items: stretch
, flex items on both lines have heights of 100px.
When you set a height on a flex item you are consuming free space. Only the remaining space gets factored into align-content: stretch
.
So in the question the first item is given height: 60px
. That leaves 140px of free space remaining in the cross axis, right? Okay, let's go with that. That space is distributed equally among both lines.
.flex-container {
display: flex;
flex-wrap: wrap;
width: 400px;
height: 200px;
border: 1px solid black;
align-items: stretch;
}
.flex-container> :nth-child(1) {
background-color: darkorchid;
height: 60px;
}
.flex-container > :nth-child(2) {
background-color: darkkhaki;
}
.flex-container > :nth-child(3) {
background-color: dodgerblue;
}
.flex-container > :nth-child(4) {
background-color: darkgoldenrod;
}
.flex-container > :nth-child(5) {
background-color: darkcyan;
}
<div class="flex-container">
<span>I am number 1</span>
<div>I am number 2</div>
<div>I am number 3</div>
<div>I am number 4</div>
<span>I am number 5</span>
</div>
At this point we should see items 2, 3 and 4 with heights of 130px, and item 5 with a height of 70px. (Item 1 doesn't participate because align-items: stretch
no longer applies. It's been overridden with a height declaration (full explanation).)
But that's not what happens. Participating items on the first row have a height of 121px, and on the second row 79px.
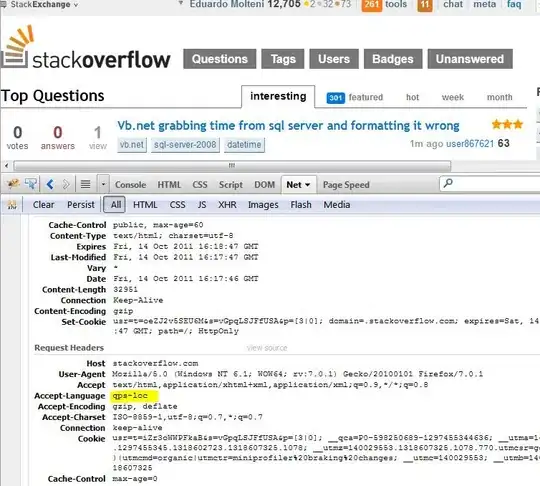
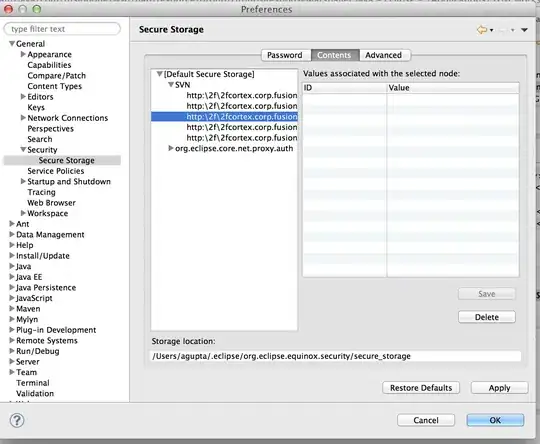
Why? Because there was less free space than originally thought. The content itself (the text) has height and consumes space. Once you factor in the height of the text (whatever it may be, I don't know), those numbers add up.
In fact, the only reason flex lines were equal height in the first example (the one with no height defined on the first item) was that all text had the same font size. If you were to change the font size of, let's say, item #5, flex lines would again have unequal heights.
.flex-container {
display: flex;
flex-wrap: wrap;
width: 400px;
height: 200px;
border: 1px solid black;
align-items: stretch;
}
.flex-container> :nth-child(1) {
background-color: darkorchid;
/* height: 60px; */
}
.flex-container > :nth-child(2) {
background-color: darkkhaki;
}
.flex-container > :nth-child(3) {
background-color: dodgerblue;
}
.flex-container > :nth-child(4) {
background-color: darkgoldenrod;
}
.flex-container > :nth-child(5) {
background-color: darkcyan;
font-size: 2em; /* demo */
}
<div class="flex-container">
<span>I am number 1</span>
<div>I am number 2</div>
<div>I am number 3</div>
<div>I am number 4</div>
<span>I am number 5</span>
</div>
More details: