I had just answered similar question here. I'll copy answer here as I think this question title is much more informative:
You can use uniform distribution with boundaries "translated" from normal to uniform space (using error function) and convert it to normal distribution using inverse error function.
import matplotlib.pyplot as plt
import numpy as np
from scipy import special
mean = 0
std = 7
min_value = 0
max_value = 15
min_in_standard_domain = (min_value - mean) / std
max_in_standard_domain = (max_value - mean) / std
min_in_erf_domain = special.erf(min_in_standard_domain)
max_in_erf_domain = special.erf(max_in_standard_domain)
random_uniform_data = np.random.uniform(min_in_erf_domain, max_in_erf_domain, 10000)
random_gaussianized_data = (special.erfinv(random_uniform_data) * std) + mean
fig, axes = plt.subplots(1, 2, figsize=(12, 6))
axes[0].hist(random_uniform_data, 30)
axes[1].hist(random_gaussianized_data, 30)
axes[0].set_title('uniform distribution samples')
axes[1].set_title('erfinv(uniform distribution samples)')
plt.show()
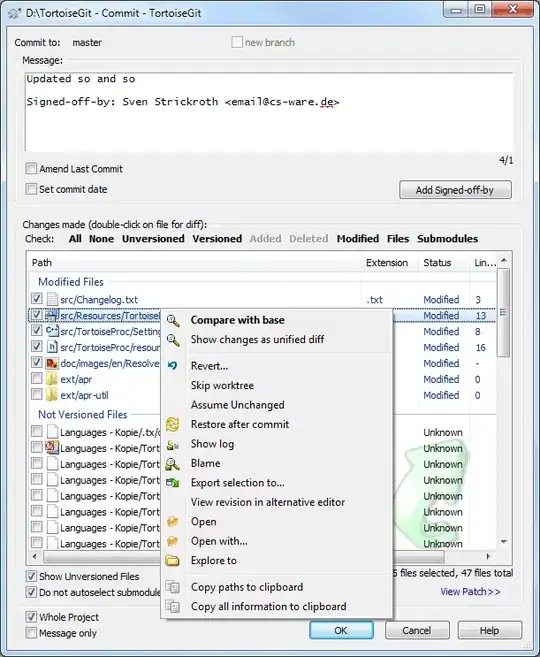