Wrong data type
Never use java.util.Date
nor java.sql.Date
classes. These classes were terribly designed, and were supplanted years ago by the modern java.time classes defined in JSR 310.
And by the way, never write code that depends implicitly on some default time zone. That puts control of your results outside your hands as a programmer.
LocalDate
To represent a date only, without a time-of-day, and without the context of a time zone or offset-from-UTC, use LocalDate
.
LocalDate localDate = LocalDate.parse( "2000-06-20" ) ; // Parses standard ISO 8601 format by default.
Exchanging LocalDate
objects with your database
Store a date-only value in a database column of a type akin to the SQL standard type DATE
. Note that various databases vary widely in their date-time handling and naming, so study your documentation closely. In MySQL 8 specifically, use its DATE
type.
Write to database.
myPreparedStatement.setObject( … , localDate );
Retrieve from database.
LocalDate localDate = myResultSet.getObject( … , LocalDate.class ) ;
If you following this approach, default time zones will be irrelevant. Your results will be consistent.
Hibernate & JPA support java.time
As for your use of Hibernate, both Hibernate and JPA were both updated years ago to support java.time. Be sure your JDBC driver complies with JDBC 4.2 or later.
Searches: Hibernate & java.time, and JPA & java.time
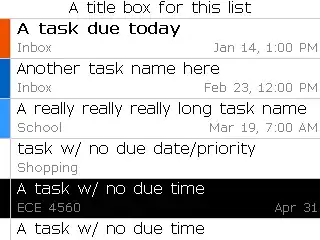
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?