The problem is that fromstring()
doesn't do what you think it does, it doesn't scale images. It can only source a data-stream into the original data-stream size. So if you're inputting 16x16
pixels of data, that's the size fromstring()
will require in order to create a image from a string/bytes stream.
This is how that function is intended to function:
from PIL import Image
print(Image.open("test.png"))
And it should give you:
<PIL.PngImagePlugin.PngImageFile image mode=RGBA size=64x64 at 0x2FCB6B8>
Where the size and modes are important. You should also use this as input vectors for fromstring()
since the size might change.
The following works perfectly fine for me:
from PIL import Image
import pygame
pygame.init()
gameDisplay = pygame.display.set_mode((800, 600))
image = Image.open("test.png")
mode = image.mode
data = image.tobytes('raw', mode)
py_image = pygame.image.fromstring(data, image.size, mode)
exit = False
while not exit:
for event in pygame.event.get():
if event.type == pygame.QUIT:
exit = True
gameDisplay.fill((255, 255, 255))
gameDisplay.blit(py_image, (10, 10))
pygame.display.update()
pygame.quit()
And produces:
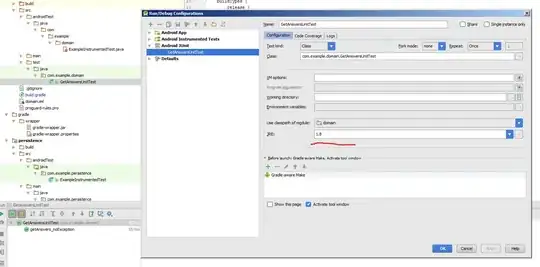
If you want to convert a small image, into a larger one. You'll first need to create a blank template in which you can read the data in to. Here I'll create a blank (white) image of 64x64
pixels, and transplant/merge a smaller 16x16
image into the bigger one at position 0, 0
.
source = Image.open("test.png")
canvas = Image.new('RGB', (64,64), (255, 255, 255))
canvas.paste(source, (0, 0))
You can then proceed to use the image as per normal. Altho, this won't scale it, it will only give you a larger canvas to work with. If you want to scale an image, you should use pygame.transform.scale or PIL scale the image.