To approximate a circle quarter using a single cubic arc what is normally done is making the middle point being exactly on the circle and using tangent starting and ending directions.
This is not formally the "best" approximation in any reasonable metric but is very easy to compute... for example the magic number for a circle quarter is 0.5522847498
. See this page for some detail...
To draw an ellipse arc instead you can just stretch the control points of the circular arc (once again not something that would qualify mathematically as "best approximation").
The generic angle case
The best arc for a generic angle under this definition (i.e. having the middle point of the bezier being on the middle of the arc) can be computed using the following method...
1. The maximum height of a symmetrical cubic bezier arc is 3/4 H where H is the height of the control point
This should be clear considering how the middle point of a bezier cubic is computed with the De Casteljau's algorithm or my answer about explicit computation of a bezier curve; see also the following picture:
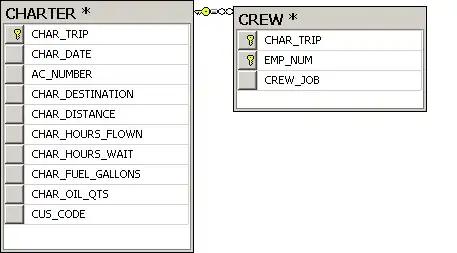
y_mid = ((0+H)/2 + (H+H)/2) / 2 = 3/4 H
2. The angle at the center for a circle cord is twice the angle at the base
See any elementary geometry text for a proof.
Finally naming
L
the distance between the extremes,
S
the (unknown) control arm of the symmetric Bezier,
2*alpha
the angle of circle to approximate we can compute that
3. S = L (2/3) (1-cos(alpha)) / (sin(alpha)**2)
This follows from the above equations and from some computation; see the following picture for a description.
