The short answer to your question is that this is not very straight-forward to do and if you're not willing to directly mess with HTML and CSS, I would recommend you don't waste time on this.
To generate the container for the checkboxes checkBoxGroupInput
uses shiny:::generateOptions
under the hood. A look at the source shows that unfortunately changing the style of individual checkboxes is not easy, because by default it applies the same class="checkbox"
to each div
(the answer pointed to in the comment only explains how to set a default style for all choices).
The only remedy I can see is to insert raw HTML directly into your UI. Even this is complicated by the fact that you "still can't apply styles (borders, etc.) directly to the checkbox element and have those styles affect the display of the HTML checkbox" as per the answer in the comment. I'm fairly new to CSS myself, but from searching through the linked references, this essentially means that you have to create the HTML checkbox from scratch and overlay the default one (apparently you can hide the default one but I haven't succeeded in that).
With all that said below you'll find a working example for your case and the output it generates beneath the code. Note that I'm using separate wrappers (.my_checkBox_red
,.my_checkBox_blue
and .my_checkBox_grey
) in each case. There is probably a way to do this more efficiently by just changing the relevant styles (colour:
, background-colour:
), but I couldn't figure this out. Also, one thing that can definitely be done quite easily to improve the below is to automate the generation of the long HTML string, but I don't have time to do this now. If you want to have a go yourself, the shiny function checkBoxGroupInput
and shiny:::generateOptions
might be a good starting point or just build your own function using sprintf
, paste
, ...
Hope this helps!
library("shiny")
ui <- fluidPage(
# The below now creates a custom css class.
tags$head(
tags$style(HTML('
.my_checkBox_red input[type="checkbox"]:before {
border: 2px solid;
color: red;
background-color: white;
content: "";
height: 15px;
left: 0;
position: absolute;
top: 0;
width: 15px;
}
.my_checkBox_red input[type="checkbox"]:checked:after {
border: 2px solid;
color: red;
background-color: #ffcccc;
content: "✓";
font-size: smaller;
vertical-align: middle;
text-align: center;
height: 15px;
left: 0;
position: absolute;
top: 0;
width: 15px;
}
.my_checkBox_blue input[type="checkbox"]:before {
border: 2px solid;
color: blue;
background-color: white;
content: "";
height: 15px;
left: 0;
position: absolute;
top: 0;
width: 15px;
}
.my_checkBox_blue input[type="checkbox"]:checked:after {
border: 2px solid;
color: blue;
background-color: #ccccff;
content: "✓";
font-size: smaller;
vertical-align: middle;
text-align: center;
height: 15px;
left: 0;
position: absolute;
top: 0;
width: 15px;
}
.my_checkBox_grey input[type="checkbox"]:before {
border: 2px solid;
color: grey;
background-color: white;
content: "";
height: 15px;
left: 0;
position: absolute;
top: 0;
width: 15px;
}
.my_checkBox_grey input[type="checkbox"]:checked:after {
border: 2px solid;
color: grey;
background-color: #e6e6e6;
content: "✓";
font-size: smaller;
vertical-align: middle;
text-align: center;
height: 15px;
left: 0;
position: absolute;
top: 0;
width: 15px;
}
'))
),
tags$div(
HTML(
'<div id="my_cbgi" class="form-group shiny-input-checkboxgroup shiny-input-container">
<label class="control-label" for="my_cbgi">Choose Something</label>
<div class="shiny-options-group">
<div class="my_checkBox_red">
<div class="checkbox">
<label>
<input type="checkbox" name="my_cbgi" value="A"/>
<span><span style="color: red;">A</span></span>
</label>
</div>
</div>
<div class="my_checkBox_red">
<div class="checkbox">
<label>
<input type="checkbox" name="my_cbgi" value="B"/>
<span><span style="color: red;">B</span></span>
</label>
</div>
</div>
<div class="my_checkBox_blue">
<div class="checkbox">
<label>
<input type="checkbox" name="my_cbgi" value="C"/>
<span><span style="color: blue;">C</span></span>
</label>
</div>
</div>
<div class="my_checkBox_grey">
<div class="checkbox">
<label>
<input type="checkbox" name="my_cbgi" value="D"/>
<span><span style="font-weight: bold;">D</span></span>
</label>
</div>
</div>
</div>
</div>'
)
),
textOutput("choice")
)
server <- function(input, output) {
output$choice = renderText({
input$my_cbgi
})
}
shinyApp(ui = ui, server = server)
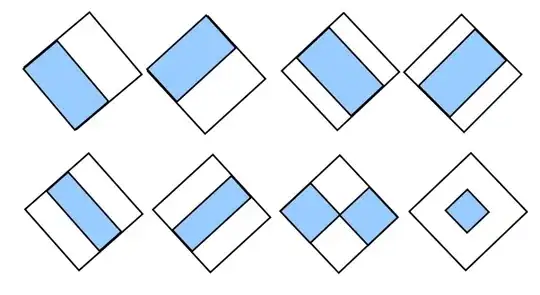