The problem is that bar plots are built out of grid::rectGrob
, and when you make the outline of a rectGrob
larger, it grows out the way. Since lines are a fixed point size, but the bars themselves aren't (as you'll see if you change the window size), there's no easy way to just shrink the rectGrob
s to compensate for this to allow for internal outlining. So this is effectively a harder problem to fix than it first appears. Of course, it's not impossible, but your three options are:
- Choose a different way to plot (like
position_dodge
)
- Achieve the effect you are looking for with a temporary hack
- Write a whole new geom to achieve the effect (or find a package that has already done this)
If this is just a one-off and you are keen to pursue a particular look for your plot, I would definitely go for option 2. Here's an example of how it could be achieved:
ggplot(dat) +
geom_col(aes(x = dx, y = num, fill = dx, color = test),
size = 3) +
scale_color_manual(values = c("orange","blue")) +
geom_segment(aes(x = 0.53, y = 100, xend = 1.465, yend = 100),
size = 3, colour = "blue") +
geom_segment(aes(x = 0.53, y = 120, xend = 1.465, yend = 120),
size = 3, colour = "orange") +
geom_segment(aes(x = 1.53, y = 40, xend = 2.465, yend = 40),
size = 3, colour = "blue") +
geom_segment(aes(x = 1.53, y = 60, xend = 2.465, yend = 60),
size = 3, colour = "orange")
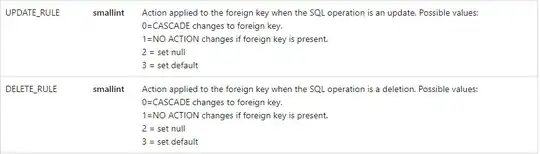