You can do that using Docotic.Pdf library. The simplest solution is to convert every source page to XObject, then scale it to landscape and draw on multiple target pages.
Here is the sample:
using System.Linq;
using BitMiracle.Docotic.Pdf;
namespace SplitToMultiplePages
{
public static class SplitToMultiplePages
{
public static void Main()
{
// NOTE:
// When used in trial mode, the library imposes some restrictions.
// Please visit http://bitmiracle.com/pdf-library/trial-restrictions.aspx
// for more information.
BitMiracle.Docotic.LicenseManager.AddLicenseData("temporary or permanent license key here");
using (var src = new PdfDocument(@"Example.pdf"))
{
// Calculate common parameters based on the first page.
// That makes sense when all pages have the same size, portrait orientation, and margins.
PdfPage srcPage = src.Pages[0];
PdfCollection<PdfTextData> words = srcPage.GetWords();
double topMargin = words[0].Position.Y;
double bottomMargin = srcPage.Height - words[words.Count - 1].Bounds.Bottom;
double scale = srcPage.Height / srcPage.Width;
const int BorderHeight = 1;
// This sample shows how to convert existing PDF content in portrait orientation to landscape
Debug.Assert(scale > 1);
using (var dest = new PdfDocument())
{
bool addDestPage = false;
double destPageY = topMargin;
for (int s = 0; s < src.PageCount; ++s)
{
if (s > 0)
{
srcPage = src.Pages[s];
words = srcPage.GetWords();
}
// skip empty pages
if (words.Count == 0)
continue;
// Get content of the source page, scale to landscape and draw on multiple pages
double textStartY = words[0].Bounds.Top;
double[] lineBottomPositions = words
.Select(w => (w.Bounds.Bottom - textStartY + BorderHeight) * scale)
.Distinct()
.ToArray();
double contentHeight = lineBottomPositions[lineBottomPositions.Length - 1];
PdfXObject xobj = dest.CreateXObject(srcPage);
double remainingHeight = contentHeight;
while (true)
{
PdfPage destPage = addDestPage ? dest.AddPage() : dest.Pages[dest.PageCount - 1];
destPage.Width = srcPage.Height;
destPage.Height = srcPage.Width;
double availableHeight = destPage.Height - destPageY - bottomMargin;
if (remainingHeight > availableHeight)
availableHeight = adjustToNearestLine(availableHeight, lineBottomPositions);
PdfCanvas destCanvas = destPage.Canvas;
destCanvas.SaveState();
destCanvas.TranslateTransform(0, destPageY);
destCanvas.AppendRectangle(new PdfRectangle(0, 0, destPage.Width, availableHeight), 0);
destCanvas.SetClip(PdfFillMode.Winding);
double y = -topMargin * scale - (contentHeight - remainingHeight);
destCanvas.DrawXObject(xobj, 0, y, xobj.Width * scale, xobj.Height * scale, 0);
destCanvas.RestoreState();
if (remainingHeight <= availableHeight)
{
// Move to next source page
addDestPage = false;
destPageY = remainingHeight + bottomMargin;
break;
}
// Need more pages in the resulting document
remainingHeight -= availableHeight;
addDestPage = true;
destPageY = topMargin;
}
}
// Optionally you can use Single Column layout by default
//dest.PageLayout = PdfPageLayout.OneColumn;
dest.Save("SplitToMultiplePages.pdf");
}
}
}
private static double adjustToNearestLine(double height, double[] lineHeights)
{
// TODO: Use binary search for better performance
for (int i = lineHeights.Length - 1; i >= 0; --i)
{
double lh = lineHeights[i];
if (height > lh)
return lh;
}
return lineHeights[0];
}
}
}
The sample produces the following result: https://drive.google.com/file/d/1ITtV3Uw84wKd9mouV4kBpPoeWtsHlB9A/view?usp=sharing
Screenshot:
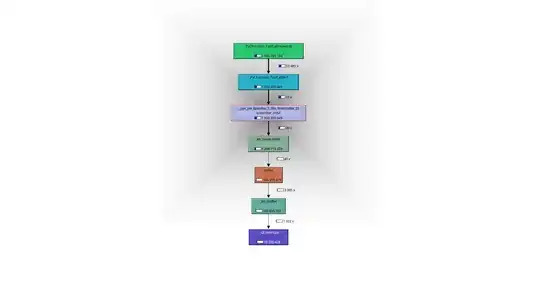
Based on your requirements you can also skip headers on all pages except the first one. Here is the sample for this case:
using System.Linq;
using BitMiracle.Docotic.Pdf;
namespace SplitToMultiplePages
{
public static class SplitToMultiplePages
{
public static void Main()
{
// NOTE:
// When used in trial mode, the library imposes some restrictions.
// Please visit http://bitmiracle.com/pdf-library/trial-restrictions.aspx
// for more information.
BitMiracle.Docotic.LicenseManager.AddLicenseData("temporary or permanent license key here");
using (var src = new PdfDocument(@"Example.pdf"))
{
// Calculate common parameters based on the first page.
// That makes sense when all pages have the same size, portrait orientation, and margins.
PdfPage srcPage = src.Pages[0];
PdfCollection<PdfTextData> words = srcPage.GetWords();
double topMargin = words[0].Position.Y;
double bottomMargin = srcPage.Height - words[words.Count - 1].Bounds.Bottom;
double scale = srcPage.Height / srcPage.Width;
const int BorderHeight = 1;
// This sample shows how to convert existing PDF content in portrait orientation to landscape
Debug.Assert(scale > 1);
using (var dest = new PdfDocument())
{
bool addDestPage = false;
double destPageY = topMargin;
for (int s = 0; s < src.PageCount; ++s)
{
if (s > 0)
{
srcPage = src.Pages[s];
words = srcPage.GetWords();
}
// skip empty pages
if (words.Count == 0)
continue;
// Get content of the source page, scale to landscape and draw on multiple pages
double textStartY = words[0].Bounds.Top;
// Skip the header line of all pages except first
if (s > 0)
{
double? firstDataRowY = words.Select(w => w.Bounds.Top).FirstOrDefault(y => y > textStartY);
if (!firstDataRowY.HasValue)
continue;
textStartY = firstDataRowY.Value;
}
double[] lineBottomPositions = words
.Select(w => (w.Bounds.Bottom - textStartY + BorderHeight) * scale)
.Distinct()
.ToArray();
double contentHeight = lineBottomPositions[lineBottomPositions.Length - 1];
PdfXObject xobj = dest.CreateXObject(srcPage);
double remainingHeight = contentHeight;
while (true)
{
PdfPage destPage = addDestPage ? dest.AddPage() : dest.Pages[dest.PageCount - 1];
destPage.Width = srcPage.Height;
destPage.Height = srcPage.Width;
double availableHeight = destPage.Height - destPageY - bottomMargin;
if (remainingHeight > availableHeight)
availableHeight = adjustToNearestLine(availableHeight, lineBottomPositions);
PdfCanvas destCanvas = destPage.Canvas;
destCanvas.SaveState();
destCanvas.TranslateTransform(0, destPageY);
destCanvas.AppendRectangle(new PdfRectangle(0, 0, destPage.Width, availableHeight), 0);
destCanvas.SetClip(PdfFillMode.Winding);
double y = -textStartY * scale - (contentHeight - remainingHeight);
destCanvas.DrawXObject(xobj, 0, y, xobj.Width * scale, xobj.Height * scale, 0);
destCanvas.RestoreState();
if (remainingHeight <= availableHeight)
{
// Move to the next source page
addDestPage = false;
destPageY = remainingHeight + bottomMargin;
break;
}
// Need more pages in the resulting document
remainingHeight -= availableHeight;
addDestPage = true;
destPageY = topMargin;
}
}
// Optionally you can use Single Column layout by default
//dest.PageLayout = PdfPageLayout.OneColumn;
dest.Save("SplitToMultiplePages.pdf");
}
}
}
private static double adjustToNearestLine(double height, double[] lineHeights)
{
// TODO: Use binary search for better performance
for (int i = lineHeights.Length - 1; i >= 0; --i)
{
double lh = lineHeights[i];
if (height > lh)
return lh;
}
return lineHeights[0];
}
}
}
The resulting file for the "skip headers" sample: https://drive.google.com/file/d/1v9lPYIposkNNgheUzz8kD3XSwMxGBJIz/view?usp=sharing