java.time
The question and the answers written at that time use java.util
Date-Time API and their formatting API, SimpleDateFormat
which was the right thing to do at that time. Java 8, released in March 2014, brought the modern date-time API. It is recommended to stop using the legacy date-time API entirely and switch to the modern Date-Time API.
Also, quoted below is a notice from the home page of Joda-Time:
Note that from Java SE 8 onwards, users are asked to migrate to java.time (JSR-310) - a core part of the JDK which replaces this project.
Your problem
You are trying to parse the date string into a java.util.Date
object and print it. A java.util.Date
object represents only the number of milliseconds since January 1, 1970, 00:00:00 GMT. When you print its object, the string returned by Date#toString
, which applies your system's timezone, is printed. Note that a date-time object holds only date-time information, not a format. A format is represented using a string you obtain by formatting the date-time object using a date-time formatting class with the desired pattern.
Modern Date-Time API
The following table shows an overview of different types available in the modern Date-Time API.
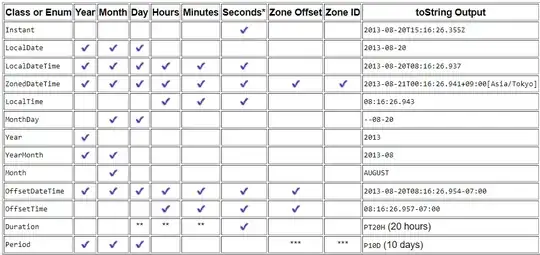
The type, LocalDate
fits your requirement. You can use different patterns for parsing and formatting using a DateTimeFormatter
. The following example uses the same pattern for parsing and formatting:
public class Main {
public static void main(String[] args) {
String strDate = "31/05/2011";
DateTimeFormatter dtf = DateTimeFormatter.ofPattern("dd/MM/uuuu", Locale.ENGLISH);
LocalDate date = LocalDate.parse(strDate, dtf);
System.out.printf("Default format: %s, Custom format: %s%n", date, date.format(dtf));
}
}
Output:
Default format: 2011-05-31, Custom format: 31/05/2011
ONLINE DEMO
Note: Here, you can use y
instead of u
but I prefer u
to y
. Also, never use DateTimeFormatter
for custom formats without a Locale.
Learn more about the modern Date-Time API from Trail: Date Time.