You should stop using the outdated and error-prone java.util
date-time API and switch to the modern date-time API.
If rfxobjet.getRv_rc_date()
returns an object of java.util.Date
, your first step should be to convert it into an object of type, Instant by calling Date#toInstant on this object i.e.
Date madate = rfxobjet.getRv_rc_date();
Instant instant = madate.toInstant();
Once you have an object of Instant
, you can convert it to any other modern date-time object as per your requirement e.g.
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.OffsetDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class Main {
public static void main(String[] args) {
Instant instant = Instant.now();
// I've used the time-zone of Europe/London as an example, you can choose any
// time-zone as per your requirement. You can also select the time-zone used by
// your JVM, ZoneId.systemDefault()
ZonedDateTime zdt = instant.atZone(ZoneId.of("Europe/London"));
OffsetDateTime odt = zdt.toOffsetDateTime();
LocalDateTime ldt = zdt.toLocalDateTime();
System.out.println(instant);
System.out.println(zdt);
System.out.println(odt);
System.out.println(ldt);
}
}
Output:
2020-06-28T16:58:27.725618Z
2020-06-28T17:58:27.725618+01:00[Europe/London]
2020-06-28T17:58:27.725618+01:00
2020-06-28T17:58:27.725618
Note: LocalDateTime
drops off information like Zone Offset and Zone ID which may be required in your business logic. Therefore, choose the appropriate class as per the table shown below (Ref):
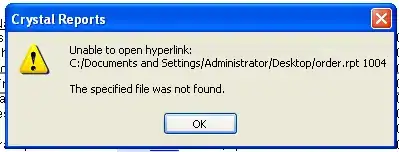
Back to your problem:
Now that you have an object of Instant
, you can get an instance of LocalDateTime
as described above i.e.
ZonedDateTime zdt = instant.atZone(ZoneId.systemDefault());
LocalDateTime ldt = zdt.toLocalDateTime();
The final step is to get the number of days between now and ldt
which you can do as follows:
LocalDateTime now = LocalDateTime.now(ZoneId.systemDefault());
long days = ChronoUnit.DAYS.between(ldt, now);
Note that I've passed ZoneId
as the parameter to LocalDateTime.now
just as the recommended practice so that you can specify the required ZoneId
as per your requirement. If you do not pass this parameter, it will use ZoneId.systemDefault()
by default.
The complete code:
Date madate = rfxobjet.getRv_rc_date();
Instant instant = madate.toInstant();
ZonedDateTime zdt = instant.atZone(ZoneId.systemDefault());
LocalDateTime ldt = zdt.toLocalDateTime();
LocalDateTime now = LocalDateTime.now(ZoneId.systemDefault());
long days = ChronoUnit.DAYS.between(ldt, now);