You can perform this using matplotlib
, pandas
and the snowflake-connector-python
Python 3.x modules installed.
You'll need to build a query that aggregates your warehouse metering history in the way you need, using the WAREHOUSE_METERING_HISTORY
account usage view or equivalent. The example provided below uses a query that aggregates each month.
With the query results in a Pandas DataFrame, you can then use pivot to format the data such that each warehouse series can appear as a line of its own.
import matplotlib.pyplot as plt
from snowflake import connector
# Establish your connection here
con = connector.connect(…)
q = """
select
warehouse_name as warehouse,
date_trunc('month', end_time)::date in_month,
sum(credits_used) credits
from snowflake.account_usage.warehouse_metering_history
where warehouse_id != 0
group by warehouse, in_month
order by warehouse, in_month;
"""
df = con.cursor().execute(q).fetch_pandas_all()
# Explicitly specify datatypes for all columns so they behave well
df['IN_MONTH'] = pd.to_datetime(df['IN_MONTH'])
tdf = df.astype({'WAREHOUSE': 'string', 'CREDITS': 'float'})
pdf = tdf.pivot(index='IN_MONTH', columns='WAREHOUSE', values='CREDITS')
pdf.plot()
plt.show()
This yields:
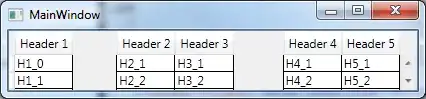
P.s. You can alternatively try the native Snowsight features in Snowflake to plot interactive charts right from its SQL editor interface:
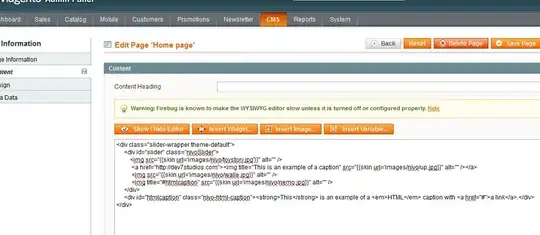