Not very clear 'what you want', but the next approach will surely improve your code, I think. It will iterate between the existing cells in column A:A and search for a match in the main folder and its sub folders. When a match is found, a hyperlink is created, displaying the folder path like ScreenTip
(when move the cursor over the cell). Please, use the next approach:
- Create the next
Private
variables on top of module keeping the code (in the declarations area):
Private iCountHyp As Long, boolFound As Boolean, arrFound As Variant
- Use the main sub, able to call the recursive
Sub
and the one creating hyperlinks for each cell value (in column A:A):
Sub testAddHypLinExistingNames()
Dim sh As Worksheet, strMainFolder As String, lastRow As Long, i As Long
strMainFolder = "\\192.168.00.01\My main folder\Documents\General\My files" 'without backslash at the end...
'strMainFolder = "C:\Teste VBA Excel\TestPDF"
Set sh = ActiveSheet 'use here your sheet
lastRow = sh.Range("A" & Rows.count).End(xlUp).row
ReDim arrFound(lastRow - 1)
iCountHyp = 0
For i = 2 To lastRow
boolFound = False
GetFold sh, strMainFolder, "pdf", sh.Range("A" & i)
Next i
End Sub
- The above
Sub
uses the next two Subs for recursive folders find and apply Hyperlink. Of course, they must be copied in the same module:
Private Sub GetFold(sh As Worksheet, strFolder As String, strExt As String, Optional rng As Range) 'recursive
Dim fld As Object, subFld As Object, oFSO As Object
If boolFound Then Exit Sub
Set oFSO = CreateObject("Scripting.FileSystemObject")
Set fld = oFSO.GetFolder(strFolder)
getFilesHyperlink sh, strFolder, strExt, IIf(Not rng Is Nothing, rng, "")
For Each subFld In fld.SubFolders
If boolFound Then Exit Sub
GetFold sh, subFld.path, strExt, IIf(Not rng Is Nothing, rng, "")
Next
End Sub
Private Sub getFilesHyperlink(sh As Worksheet, strFolder As String, strExt As String, Optional rng As Range)
Dim MyFolder As Object, f As Object, oFSO As Object, boolF As Boolean, El As Variant
Set oFSO = CreateObject("Scripting.FileSystemObject")
Set MyFolder = oFSO.GetFolder(strFolder)
For Each f In MyFolder.Files
If LCase(oFSO.GetExtensionName(f.Name)) = "pdf" Then
If Not rng Is Nothing Then
If f.Name = rng.Value Then
For Each El In arrFound
If El = rng.Value Then boolF = True: Exit For
Next
If Not boolF Then
sh.Hyperlinks.aDD Anchor:=rng, address:=strFolder & "\" & f.Name, _
ScreenTip:=strFolder, TextToDisplay:=rng.Value
boolFound = True: arrFound(iCountHyp + 1) = rng.Value: iCountHyp = iCountHyp + 1
rng.Offset(, 1).Value = "OK"
Exit Sub
End If
End If
Else
sh.Hyperlinks.aDD Anchor:=sh.Range("A" & iCountHyp), address:=strFolder & "\" & f.Name, _
ScreenTip:=strFolder, TextToDisplay:=f.Name
iCountHyp = iCountHyp + 1
End If
End If
Next
End Sub
The result will look as in the picture:
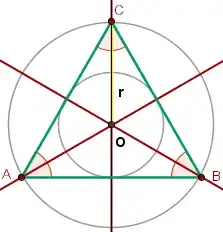