If you want to get the login user in the sever side. You should make the service as scope and then you could inject the AuthenticationStateProvider
into service.
Then you could get the current login user.
Details, you could refer to below codes:
public class WeatherForecastService
{
private readonly AuthenticationStateProvider _authenticationStateProvider;
public WeatherForecastService(AuthenticationStateProvider authenticationStateProvider) {
_authenticationStateProvider = authenticationStateProvider;
}
public string GetCurrentUserName() {
var provider= _authenticationStateProvider.GetAuthenticationStateAsync();
return provider.Result.User.Identity.Name;
}
}
As far as I know, if you want to get the current login user, you could try to use AuthenticationStateProvider service.
The built-in AuthenticationStateProvider service obtains authentication state data from ASP.NET Core's HttpContext.User.
You could inject the AuthenticationStateProvider AuthenticationStateProvider
and then use AuthenticationStateProvider.GetAuthenticationStateAsync
to get the user state, at last ,you could use user.Identity.Name
to get the current user name.
More details ,you could refer to below codes:
@page "/counter"
@using Microsoft.AspNetCore.Components.Authorization
@inject AuthenticationStateProvider AuthenticationStateProvider
<h1>Counter</h1>
<p>Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
<hr />
<button class="btn btn-primary" @onclick="GetUserName">Click me</button>
<p>Current Login User = @Username</p>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
private string Username = string.Empty;
private async Task GetUserName()
{
var authState = await AuthenticationStateProvider.GetAuthenticationStateAsync();
var user = authState.User;
Username = user.Identity.Name;
}
}
Result:
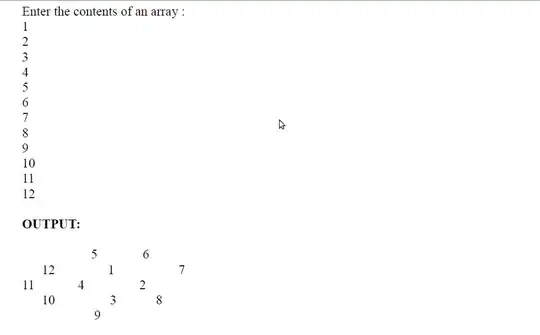