Just for you, I've quickly wrote this. This isn't the most efficient way of doing this also you can implement more validations like for decimal numbers or non-negative numbers. Also you can make TexBox
IsReadOnly = true
to make sure user don't input any non-numeric values.
You can do a lot of the things ! this is just to give you an idea.
XAML
<StackPanel Orientation="Horizontal" Width="200" VerticalAlignment="Center" HorizontalAlignment="Center">
<TextBox x:Name="NumberBox" VerticalAlignment="Center" Width="120" Text="0"></TextBox>
<StackPanel Orientation="Vertical" VerticalAlignment="Center"
Margin="2 0 0 0" Spacing="1">
<Button x:Name="UpBtn" Width="50" Height="25" Click="IncrementDecrement">▲</Button>
<Button x:Name="DownBtn" Width="50" Height="25" Click="IncrementDecrement">▼</Button>
</StackPanel>
</StackPanel>
Back-End Code (non MVVM WAY)
private void IncrementDecrement(object sender, RoutedEventArgs e)
{
var senderBtn = sender as Button;
var value = NumberBox.Text.Trim();
if (string.IsNullOrEmpty(value))
{
value = 0.ToString();
}
bool success = Int32.TryParse(value, out var number);
if (!success)
{
// show error
return;
}
NumberBox.Text = senderBtn.Name == "UpBtn" ? (++number).ToString() : (--number).ToString();
}
Result
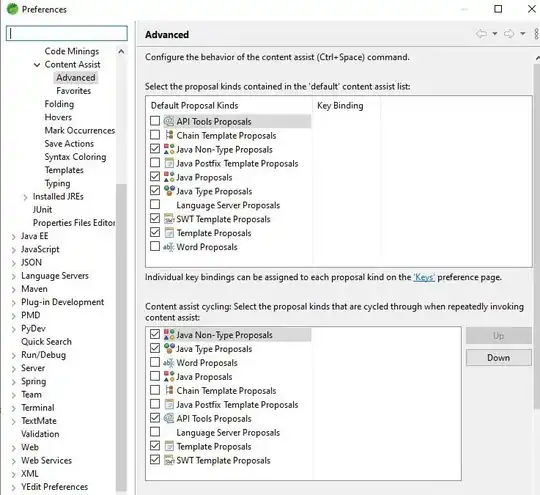