Use curly bracketed numbers:
M.{5}d //M, then 5 of anything, then d
M.{5,7}d //M, then between 5 and 7 of anything, then d
M.{,5}d //M, then upto 5 of anything, then d
M.{5,}d //M, then at least 5 of anything, then d
In regex {x,y} means "between x and y of the previous character/character class". If x is not specified, 0 is assumed. If y is not specified, infinity is assumed. If the comma is not specified, the single number means "exactly this amount of characters". The only thing that probably won't work out in all engines (maybe in any engines) is not specifying either x or y. For example, in Python {,}
just matches bracket comma bracket, it's not a range between 0 and infinity
PS: If you think about it, these are equivalent:
//zero or more of previous
.*
.{0,}
//one or more of previous
.+
.{1,}
You can also apply the ? Pessimistic modifier to a ranged quantifier. Both these mean "match an M, then at least one but as few chars as possible, then a d", i.e.. they will match the "Murd" in "Murdered"
M.+?
M.{1,}?d
Edit 2:
As per Seb's comment, because Murdered contains two d characters, it achieves a match with M.{1,5}d
:
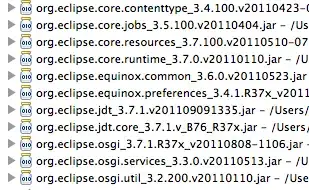
You'll have to do something else, like edit the regex so it finishes with a \b
to match the end of the word. This will prevent it matching the partial murd, but still allow a match on mud mind meld marred etc
Edit 3:
.
is frequently a poor choice of character to use - "match anything" is often more than you want. If you want to match only word characters, or everything that isn't whitespace, you can use those character classes instead:
M\w{1,5}d
M\S{1,5}d
Always try to find ways of avoiding .
especially if you're seeing problems of more things matching than you want