Actually, if you read the question carefully, the number of integers on each line is given.
The next line contains an integer p (0 ≤ p ≤ n) at first, then follows p distinct integers a 1, a 2, ..., a p (1 ≤ a i ≤ n). These integers denote the indices of levels Little X can pass. The next line contains the levels Little Y can pass in the same format. It's assumed that levels are numbered from 1 to n.
p = Number of integers on each line.
Have a look at the following code which has Accepted code verdict on Codeforces:
#include<iostream>
#include<set>
#include<iterator>
int main(){
int N;
std::cin>>N;
std::set<int> uniqueNumbers;
int t1, t2;
int x;
std::cin>>t1;
for(int i=0;i<t1;i++){
std::cin>>x;
uniqueNumbers.insert(x);
}
std::cin>>t2;
for(int i=0;i<t2;i++){
std::cin>>x;
uniqueNumbers.insert(x);
}
std::cout<< ((uniqueNumbers.size()==N) ? "I become the guy." : "Oh, my keyboard!");
return 0;
}
Verdict:
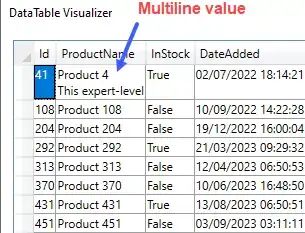