So you asked to read text from input and calculate letter & word count:
#include <stdio.h>
#include <ctype.h>
int main()
{
char a[1000] = {0};
//read a line from input
scanf("%[^\n]s", a);
int word_count = 0;
int letter_count = 0;
int idx = 0;
// go through the line
while (a[idx]){
//skip spaces
while(a[idx] && isspace(a[idx]))
idx++;
// if next char is a letter => we found a word
if (a[idx])
word_count++;
//skip the word, increment number of letters
while (a[idx] && !isspace(a[idx])){
letter_count++;
idx++;
}
}
printf("word count = %d letter count = %d", word_count, letter_count);
return 0;
}
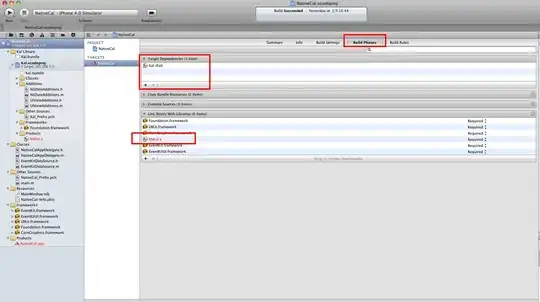
EDIT : display line count also
#include <stdio.h>
#include <ctype.h>
int main()
{
char a[1000] = {0};
//read everyting from input until character '0' is found
scanf("%[^0]s", a);
int word_count = 0;
int letter_count = 0;
int sent_count = 0;
int idx = 0;
// go through the lines
while (a[idx]){
//skip spaces
//newline is also a space, check and increment counter if found
while(a[idx] && isspace(a[idx])){
if (a[idx] == '\n')
sent_count++;
idx++;
}
// if next char is a letter => we found a word
if (a[idx])
word_count++;
//skip the word, increment number of letters
while (a[idx] && !isspace(a[idx])){
letter_count++;
idx++;
}
}
printf("word count = %d letter count = %d line count = %d", word_count, letter_count, sent_count);
return 0;
}
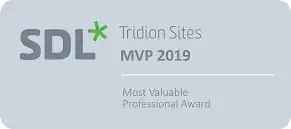
Here's another way:
#include <stdio.h>
#include <string.h>
int main()
{
char a[1000] = {0};
int word_count = 0;
int letter_count = 0;
while (1){
scanf("%s", a);
// break when word starts with '0'
if (a[0] == '0')
break;
word_count++;
letter_count += strlen(a);
}
printf("word count = %d letter count = %d", word_count, letter_count);
return 0;
}
This way reads input until word starting with character '0' is found
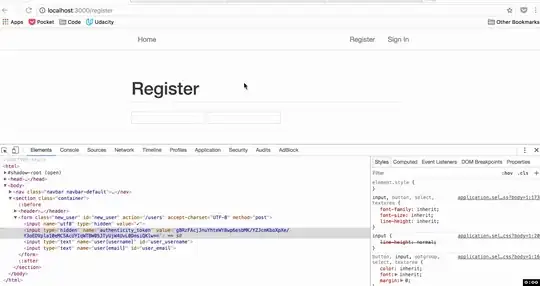
// go through the line
while (a[idx]){
while(a[idx] && isspace(a[idx]))
idx++; – Pike1406 Jul 10 '20 at 15:57