Access modifiers in swift have been charged a few times during the past few releases.
For now, let me suggest a code sample instead of explanation first:
All code in one file:
fileprivate class Foo {
private var someVariablePrivate: Int?
fileprivate var someVariableFileprivate: Int?
private func makeBar() { }
fileprivate func makeFoo() { }
}
extension Foo {
func bar() {
let value = someVariablePrivate // OK
let value2 = someVariableFileprivate // OK
}
}
private class Foofoo: Foo {
override func makeFoo() {
// OK
}
override func makeBar() {
// NOK, because private
}
}
class Bar {
func foo() {
let object = Foo()
let value = object.someVariableFileprivate // OK
let value2 = object.someVariablePrivate // NOK - because it private to that class
}
}
Previously, private restrict access only to type definition, but within few lates version of Swift, it has been modified and updated, so even extension of same type can use private variables
As Apple mention:
File-private access restricts the use of an entity to its own defining
source file. Use file-private access to hide the implementation
details of a specific piece of functionality when those details are
used within an entire file.
Private access restricts the use of an entity to the enclosing
declaration, and to extensions of that declaration that are in the
same file. Use private access to hide the implementation details of a
specific piece of functionality when those details are used only
within a single declaration.
we could compare a bit this modifiers:
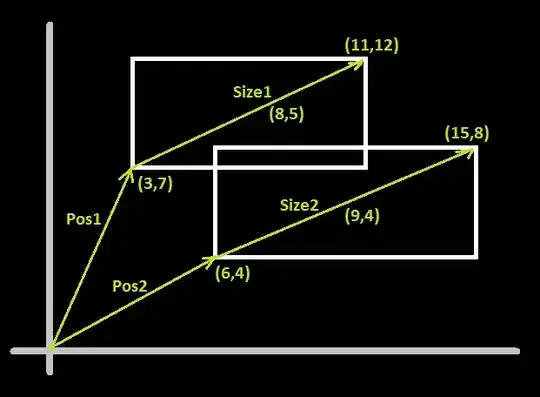
why do we ned both of them:
You may, however, want to mark some parts of your code as file private
or private in order to hide their implementation details from other
code within the app’s module.