If you want to move all repositry from particularly region to another account (Destination account) then use below script.
- It will list all repo from Account A
- Pull an image from an account A one by one
- Create Repo in Account B
- Tag image
- push image to account B
#!/bin/bash
TARGET_ACCOUNT_REGION="us-west-2"
DESTINATION_ACCOUNT_REGION="us-west-2"
DESTINATION_ACCOUNT_BASE_PATH="123456.dkr.ecr.$DESTINATION_ACCOUNT_REGION.amazonaws.com/"
REPO_LIST=($(aws ecr describe-repositories --query 'repositories[].repositoryUri' --output text --region $TARGET_ACCOUNT_REGION))
REPO_NAME=($(aws ecr describe-repositories --query 'repositories[].repositoryName' --output text --region $TARGET_ACCOUNT_REGION))
for repo_url in ${!REPO_LIST[@]}; do
echo "star pulling image ${REPO_LIST[$repo_url]} from Target account"
docker pull ${REPO_LIST[$repo_url]}
# Create repo in destination account, remove this line if already created
aws ecr create-repository --repository-name ${REPO_NAME[$repo_url]}
docker tag ${REPO_LIST[$repo_url]} $DESTINATION_ACCOUNT_BASE_PATH/${REPO_NAME[$repo_url]}
docker push $DESTINATION_ACCOUNT_BASE_PATH/${REPO_NAME[$repo_url]}
done
Make sure you already obtain login token for both account or add these command in the script.
aws ecr get-login-password --region $TARGET_ACCOUNT_REGION | docker login --username AWS --password-stdin ${REPO_LIST[$repo_url]}
# destination account login, make sure profile set for accoutn destination
aws ecr get-login-password --region $DESTINATION_ACCOUNT_REGION --profile destination_account | docker login --username AWS --password-stdin ${REPO_LIST[$repo_url]}
aws-cli-cheatsheet
Or you can use one of them
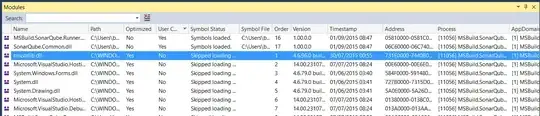
Amazon ECR uses registry settings to configure features at the
registry level. The private registry settings are configured
separately for each Region. Currently, the only registry setting is
the replication setting, which is used to configure cross-Region and
cross-account replication of the images in your repositories