We can check the registry for the status.
- Add Microsoft.Win32.Registry from NuGet.
- Check for registry status.
`
using Microsoft.Win32;
...
static bool IsWindowsVirusProtectionEnabledAsync()
{
var subKey = Registry.LocalMachine.OpenSubKey(
@"SOFTWARE\Microsoft\Windows Advanced Threat Protection\Status", false);
return (subKey != null &&
subKey.GetValueNames().Contains("OnboardingState") &&
subKey.GetValue("OnboardingState").Equals(1));
}
Also please read this. There might be other keys you might need to watch out for such as DisableAntiSpyware and DisableAntiVirus. I am not 100% sure about this.
Edit 2:
I was unable to find a shortcut or cmd line to open "Virus & Threat Window", however, I can manually start it:
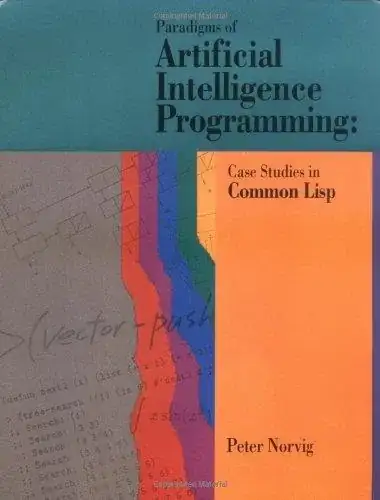
So, a bit of a hack method would be to start it manually by emulating the keyboard to start the "Virus & Threat Window": (it is a bit of a hack but it does work for me).
[DllImport("user32.dll", SetLastError = true)]
static extern void keybd_event(byte bVk, byte bScan, int dwFlags, int dwExtraInfo);
const int KEYEVENTF_KEYDOWN = 0x0000; // New definition
const int KEYEVENTF_EXTENDEDKEY = 0x0001; //Key down flag
const int KEYEVENTF_KEYUP = 0x0002; //Key up flag
const int VK_ESCAPE = 0x1B;
const int VK_0 = 0x30;
const int VK_1 = 0x31;
const int VK_2 = 0x32;
const int VK_3 = 0x33;
const int VK_4 = 0x34;
const int VK_5 = 0x35;
const int VK_6 = 0x36;
const int VK_7 = 0x37;
const int VK_8 = 0x38;
const int VK_9 = 0x39;
const int VK_A = 0x41;
const int VK_B = 0x42;
const int VK_C = 0x43;
const int VK_D = 0x44;
const int VK_E = 0x45;
const int VK_F = 0x46;
const int VK_G = 0x47;
const int VK_H = 0x48;
const int VK_I = 0x49;
const int VK_J = 0x4A;
const int VK_K = 0x4B;
const int VK_L = 0x4C;
const int VK_M = 0x4D;
const int VK_N = 0x4E;
const int VK_O = 0x4F;
const int VK_P = 0x50;
const int VK_Q = 0x51;
const int VK_R = 0x52;
const int VK_S = 0x53;
const int VK_T = 0x54;
const int VK_U = 0x55;
const int VK_V = 0x56;
const int VK_W = 0x57;
const int VK_X = 0x58;
const int VK_Y = 0x59;
const int VK_Z = 0x5A;
const int VK_LCONTROL = 0xA2; //Left Control key code
const int VK_SHIFT = 0x10;
const int VK_SPACE = 0x20;
const int VK_RETURN = 0x0D;
static void StartVirusAndThreatProtectionUI()
{
Console.WriteLine("Starting...");
keybd_event(VK_LCONTROL, 0, KEYEVENTF_KEYDOWN, 0);
keybd_event(VK_ESCAPE, 0, KEYEVENTF_KEYDOWN, 0);
keybd_event(VK_ESCAPE, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_LCONTROL, 0, KEYEVENTF_KEYUP, 0);
Thread.Sleep(1000); // in case the computer is slow
keybd_event(VK_V, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_V, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_I, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_I, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_R, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_R, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_U, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_U, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_S, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_S, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_SPACE, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_SPACE, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_SHIFT, 0, KEYEVENTF_KEYDOWN, 0);
keybd_event(VK_7, 0, KEYEVENTF_KEYDOWN, 0);
keybd_event(VK_7, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_SHIFT, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_SPACE, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_SPACE, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_T, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_T, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_H, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_H, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_R, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_R, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_E, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_E, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_A, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_A, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_T, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_T, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_SPACE, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_SPACE, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_P, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_P, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_R, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_R, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_O, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_O, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_T, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_T, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_E, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_E, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_C, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_C, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_T, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_T, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_I, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_I, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_O, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_O, 0, KEYEVENTF_KEYUP, 0);
keybd_event(VK_N, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_N, 0, KEYEVENTF_KEYUP, 0);
Thread.Sleep(1000); // In case the search takes time...
keybd_event(VK_RETURN, 0, KEYEVENTF_KEYDOWN, 0); keybd_event(VK_RETURN, 0, KEYEVENTF_KEYUP, 0);
Console.WriteLine("Hopefully Done.");
}