Thanks to j_4321 suggestion and the code from the response in the linked question, I came up with the following solution.
It is not perfect, as I still need to manually adjust the values for different sized plots. It also needs automatic aspect, which skews the heatmap somewhat:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
def draw_brace(ax, span, text, axis):
"""Draws an annotated brace on the axes."""
# axis could be reversed
xx = ax.get_xlim()
xmin = np.min(xx)
xmax = np.max(xx)
yy = ax.get_ylim()
ymin = np.min(yy)
ymax = np.max(yy)
xspan = xmax - xmin
yspan = ymax - ymin
if axis=="y":
tspan = yspan
ospan = xspan
omin = xmin
else:
ospan = yspan
omin = ymin
tspan = xspan
amin, amax = span
span = amax - amin
resolution = int(span/tspan*100)*2+1 # guaranteed uneven
beta = 300./tspan # the higher this is, the smaller the radius
x = np.linspace(amin, amax, resolution)
x_half = x[:resolution//2+1]
y_half_brace = (1/(1.+np.exp(-beta*(x_half-x_half[0])))
+ 1/(1.+np.exp(-beta*(x_half-x_half[-1]))))
y = np.concatenate((y_half_brace, y_half_brace[-2::-1]))
y = omin + (.05*y - .01)*ospan # adjust vertical position
#ax.autoscale(False)
if axis == "y":
ax.plot(-y +1 , x, color='black', lw=1)
ax.text(0.8+ymin+.07*yspan, (amax+amin)/2., text, ha='center', va='center')
else:
ax.plot(x, y, color='black', lw=1)
ax.text((amax+amin)/2.,ymin+.07*yspan, text, ha='center', va='center')
arr = np.array([[3,4],[2,3.5],[10,11],[9,10]])
fig = plt.figure()
gs = fig.add_gridspec(nrows=1, ncols=2, wspace=0,width_ratios=[1,4])
ax2 = fig.add_subplot(gs[:, 1])
ax2.imshow(arr)
ax2.set_title("example plot")
ax2.set_yticklabels([])
ax2.set_yticks([])
ax2.set_xticklabels([])
ax2.set_aspect('auto')
ax1 = fig.add_subplot(gs[:, 0], sharey=ax2)
ax1.set_xticks([])
ax1.set_xticklabels([])
ax1.set_aspect('auto')
ax1.set_xlim([0,1])
ax1.axis('off')
draw_brace(ax1, (0, 1), 'group1',"y")
draw_brace(ax1, (2, 3), 'group2',"y")
fig.subplots_adjust(wspace=0, hspace=0)
This creates the following plot:
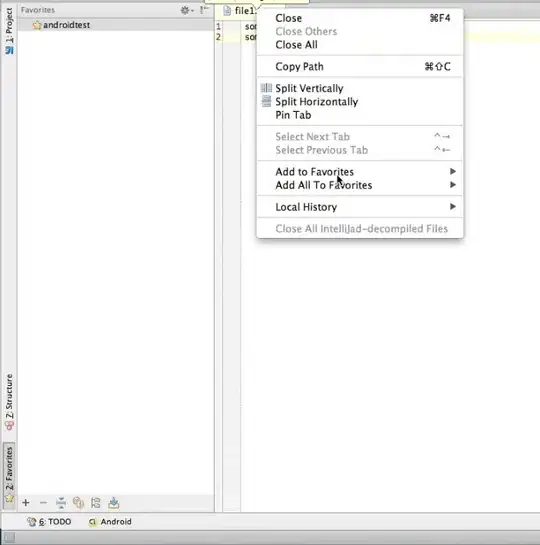