The first snippet of OP:
class c
{
public:
int x = 0;
friend constexpr auto operator +( int const left,
c const & a ) noexcept
{
return c{ left + x };
}
};
It shows a free function (operator) that is inline defined in class c
as friend
.
This is not a member function of class c
although it may look so on the first glance.
(If it would be a member function it would be a ternary operator+ but there doesn't exist one in C++ nor can it be overloaded.)
The second snippet of OP:
friend constexpr auto c::operator +( int const left,
c const & a ) noexcept
{
return c{ left + x };
}
It is wrong for two reasons:
friend
doesn't make sense. (friend
to what?)
If it's a free function - scope c::
doesn't make sense.
The friendship of functions cannot be declared outside of the resp. class.
If this would be allowed everybody could declare friendship of functions everywhere which accesses the private
members of classes. This would make the sense of private
members somehow useless.
(Imagine a safe with an electronic lock and a key pad where the password is written on the door.)
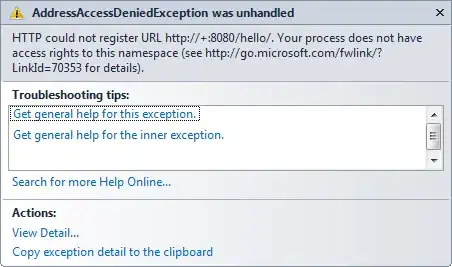
(Original image by Binarysequence - Own work, CC BY-SA 4.0, Link)
Nevertheless, friend
functions (and operators) can be defined non-inline, of course.
The following sample shows how:
#include <iostream>
// forward declaration of class c
class c;
// forward declaration of operator +
constexpr auto operator+(int, c const&) noexcept;
// the class c
class c {
// make operator + a friend to grant access to private members
friend constexpr auto operator+(int, c const&) noexcept;
private:
int x;
public:
c() = default;
constexpr c(int x): x(x) { }
int get() const { return x; }
};
// the operator +
constexpr auto operator+(int lhs, c const &rhs) noexcept
{
return c(lhs + rhs.x);
}
// show it in action:
#define DEBUG(...) std::cout << #__VA_ARGS__ << ";\n"; __VA_ARGS__
int main()
{
DEBUG(c a(123));
DEBUG(std::cout << (234 + a).get() << '\n');
}
Output:
c a(123);
std::cout << (234 + a).get() << '\n';
357
Live Demo on coliru
This is similar when applied to templates (although the syntax is a bit more tricky):
#include <iostream>
// forward declaration of class c
template <typename T>
class c;
// forward declaration of operator +
template <typename T>
constexpr auto operator+(int, c<T> const&) noexcept;
// the class c
template <typename T>
class c {
friend constexpr auto operator+<>(int, c<T> const&) noexcept;
private:
T x;
public:
c() = default;
constexpr c(T x): x(x) { }
int get() const { return x; }
};
// the operator +
template <typename T>
constexpr auto operator+(int lhs, c<T> const &rhs) noexcept
{
return c(lhs + rhs.x);
}
// show it in action:
#define DEBUG(...) std::cout << #__VA_ARGS__ << ";\n"; __VA_ARGS__
int main()
{
DEBUG(c<int> a(123));
DEBUG(std::cout << (234 + a).get() << '\n');
}
Output:
c<int> a(123);
std::cout << (234 + a).get() << '\n';
357
Live Demo on coliru
More about inline friend functions: