One way to do this could be to have the JButton
on the same class as the JTextField
, for a simple GUI as the one you have this is doable.
But we know there are times that this isn't possible so another way that you could have this done up to Java 8 is using the Observer
pattern, as shown in this example. After Java 9, Observer
and Observable
are deprecated so you should use PropertyChangeEvent
and PropertyChangeListener
from java.beans
package1.
So, if you're not using Java 9 or higher and don't care about the caveats of Observer
and Observable
then you could have a sample code like this one:
EnableButtonInOtherClass
import java.awt.BorderLayout;
import java.util.Observable;
import java.util.Observer;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
public class EnableButtonInOtherClass implements Observer {
private JFrame frame;
private OtherClass pane;
private JButton button;
private ObservableField observableField;
public static void main(String[] args) {
SwingUtilities.invokeLater(new EnableButtonInOtherClass()::createAndShowGUI);
}
private void createAndShowGUI() {
frame = new JFrame(getClass().getSimpleName());
observableField = new ObservableField();
observableField.addObserver(this);
pane = new OtherClass(observableField);
button = new JButton("Click me!");
button.setEnabled(false);
frame.add(pane);
frame.add(button, BorderLayout.SOUTH);
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
@Override
public void update(Observable o, Object arg) {
System.out.println("Text is a digit: " + arg);
button.setEnabled((boolean) arg);
}
}
OtherClass
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
@SuppressWarnings("serial")
public class OtherClass extends JPanel implements DocumentListener {
private JTextField field;
private ObservableField observableField;
public OtherClass(ObservableField observableField) {
field = new JTextField(10);
add(field);
this.observableField = observableField;
field.getDocument().addDocumentListener(this);
}
@Override
public void changedUpdate(DocumentEvent e) {
// TODO Auto-generated method stub
}
@Override
public void insertUpdate(DocumentEvent e) {
updateState();
}
@Override
public void removeUpdate(DocumentEvent e) {
updateState();
}
private void updateState() {
String patternString = "[0-9]+";
Pattern pattern = Pattern.compile(patternString);
Matcher matcher = pattern.matcher(field.getText());
observableField.updateState(matcher.matches());
}
}
ObservableField
import java.util.Observable;
public class ObservableField extends Observable {
public void updateState(boolean state) {
setChanged();
notifyObservers(state);
}
}
The above example makes use of the ObservableField
class to handle the Observable
class, that has an update method that notifies the observers (EnableButtonInOtherClass
) that there has been a change every time you type into the JTextField
on OtherClass
and based on the matcher.matches()
value determines if the JButton
should be enabled or not.
But again, for a simple UI as the one you have it's easier to move the JButton
to the same file and / or on JButton
's ActionListener
evaluate what's inside the JTextField
rather than enable / disable the JButton
.
Here are some screenshots of how the program looks like.
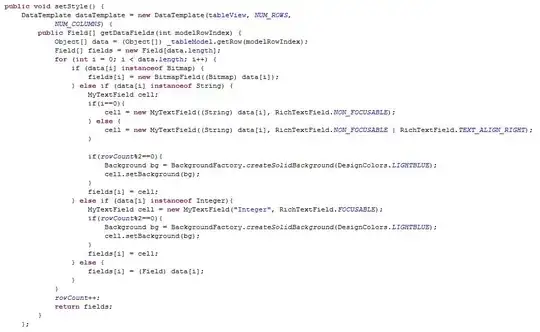
1Taken from this answer