Probably your dataframe doesn't contain the information you're expecting. Or maybe the F4
part has a completely different range? If e.g. the values of Km
, Liquid
are 10 times smaller, they would get reduced to just a line near zero.
You could print out df2['Counts'][471:494]
and df2['Counts'][495:]
to see how the values look like. The code below adds a scatter plot to see all the points.
Some remarks:
- Slicing as in
array[a:b]
goes from a
till b-1
(similar to python's range function). So you might want to use data = [df2['Counts'][0:471], df2['Counts'][471:495],df2['Counts'][495:]]
or so.
- It is recommended not to mix the
ax.
and plt.
way of calling matplotlib functions.
- Usually it is better to call the
xticks
functions after creating the main plot, as many functions try to set their own ticks.
- When setting the
xticklabels
, it is recommended to set the corresponding xticks
at the same time. In this case, the ticks are numbered internally as 1, 2, 3
.
Here is some example code showing how things could fit together. To help debugging, a scatter plot is added to see where all the points really are.
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
df2 = pd.DataFrame({'Counts': np.random.normal(0.02, 0.002, 513)})
data = [df2['Counts'][0:471], df2['Counts'][471:495], df2['Counts'][495:]]
fig1, ax4 = plt.subplots()
ax4.boxplot(data)
for i in range(3):
ax4.scatter(np.repeat([i+1], len(data[i])), data[i])
ax4.set_title('Furter vs Krum serum')
ax4.set_xticks([1, 2, 3])
ax4.set_xticklabels(['F4', 'Km', 'Liquid'])
plt.show()
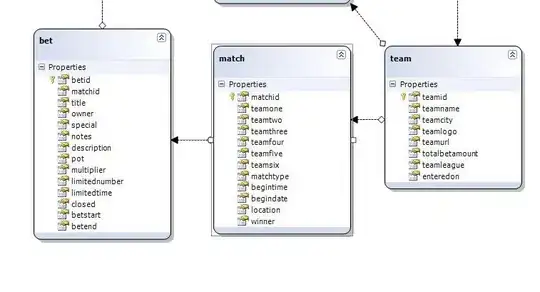