Personal Access Token
secrets.GITHUB_TOKEN
is defined by default but it is only sufficient to deploy to the current repository.
To make it work across repositories you'll need to define a new Personal Access Token in:
Select write:packages
for the scope and all the repo
scopes should be automatically selected for you.
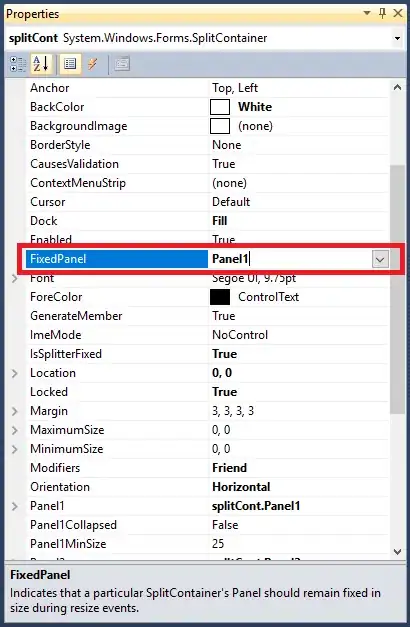
Repository / Organisation secrets
Next, define a secret in your organisation or each of the repositories you need to publish packages from.
Give it a name (i.e. DEPLOY_GITHUB_TOKEN
) and set its value to the Personal Access Token created in the previous step.
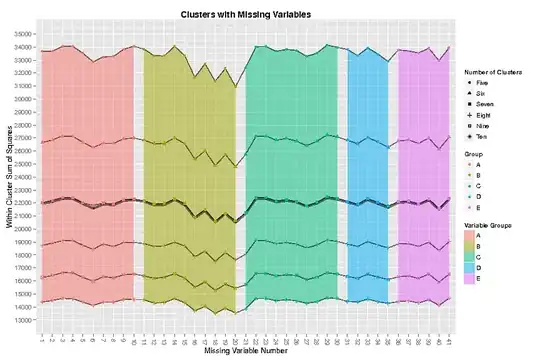
Repository secrets are defined in repository Settings
> Secrets
. There's a similar section for the organisation.
GitHub Action
Finally, make sure you pass your Personal Access Token to the deployment step as an environment variable called GITHUB_TOKEN
.
In the example below, it's set to the value of the DEPLOY_GITHUB_TOKEN
secret defined in the previous step.
name: Build
on:
release:
types: [created]
jobs:
build:
name: Build & Deploy
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 1.8
uses: actions/setup-java@v1
with:
java-version: 1.8
- name: Build with Maven
run: mvn --batch-mode --update-snapshots install
- name: Deploy to GitHub
run: mvn --batch-mode -DskipTests -DuseGitHubPackages=true deploy
env:
GITHUB_TOKEN: ${{ secrets.DEPLOY_GITHUB_TOKEN }}
Since I used a dedicated Maven profile for the GitHub package repository distribution management, I also activated it with -DuseGitHubPackages=true
.
Maven profile
In the profile example below, I configured distribution management to use the external/shared repository vlingo/vlingo-platform
just like suggested in @Danny Varod's answer.
<!-- pom.xml -->
<project>
<!-- ... -->
<profiles>
<profile>
<id>github</id>
<activation>
<property>
<name>useGitHubPackages</name>
<value>true</value>
</property>
</activation>
<distributionManagement>
<repository>
<id>github</id>
<name>GitHub Packages</name>
<url>https://maven.pkg.github.com/vlingo/vlingo-platform</url>
</repository>
</distributionManagement>
</profile>
</profiles>
</project>
Cross posted from: https://dev.to/jakub_zalas/how-to-publish-maven-packages-to-a-single-github-repository-3lkc
A working example can be found in vlingo repositories: https://github.com/vlingo/vlingo-platform/packages