Check this,
void rotate(char matrix[][10]){
char * pMatrix = &matrix[0][0];
for(int j = 0; j < 10; j ++){
for(int i = 9; i >= 0; i --){
*pMatrix++ = matrix[i][j];
}
}
}
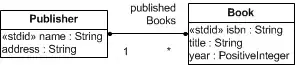
One dimensional array:
Consider an array a of 4 integers as a[4]
.
Basic rule is both a
and &a
will point to same location.But they aren't pointing to the same type.
(1) &a
will point to the entire array which is an int[]
. The pointer type is int(*)[]
(2) a
, when it decays to a pointer, will point to the first element of the array which is an int
. The pointer type is int *
Now consider two-dimensional array.
Two dimensional array
Consider an array containing two 1D arrays, with each of them having two elements; a[2][2]
.
Since the number of dimensions increases, we have one more level of hierarchy i.e. &a
, a
and *a
will point to the same location, but they aren't pointing to the same type.
(1) &a
will point to the entire array which is int[][]
. The pointer type is int(*)[][].
(2) a
, when it decays to a pointer, will point to the first element of the 2D array which is an int[]
. The pointer type is int(*)[]
(3) By using *a
, we are de-referencing the pointer to a 1D array. Hence we will have a int *
pointing to the first integer value of the 2D array.
Now considering your case,
(1) In your case is like the first case where you are just using 1 D array, and the one which I suggested as &matrix[0][0]
, the pointer points to the entire array. Hope this is clear now.
(2) Also, you need not pass the first dimension in the function.