According to your description, you want to have the same file structure in the folder and all of its sub-directories.
You can put the Copy Directory method in your code and define two string variables. One is the source address and the other is the target address.
This method will copy all the files in the source address folder to the price inquiry folder of the target address.
So you can have the same file structure and all files in the folder.
Here is a code example you can refer to:
private void Button_Click(object sender, RoutedEventArgs e)
{
string sourcePath = @"C:\Users\source\repos\MyProject";
string destPath = @"C:\Users\Desktop\New folder";
CopyDirectory(sourcePath,destPath);
}
public static void CopyDirectory(string srcPath, string destPath)
{
try
{
DirectoryInfo dir = new DirectoryInfo(srcPath); FileSystemInfo[] fileinfo = dir.GetFileSystemInfos();
foreach (FileSystemInfo i in fileinfo)
{
if (i is DirectoryInfo)
{
if (!Directory.Exists(destPath + "\\" + i.Name))
{
Directory.CreateDirectory(destPath + "\\" + i.Name);
}
CopyDirectory(i.FullName, destPath + "\\" + i.Name);
}
else
{
File.Copy(i.FullName, destPath + "\\" + i.Name, true);
}
}
}
catch (Exception e)
{
throw;
}
}
Result:
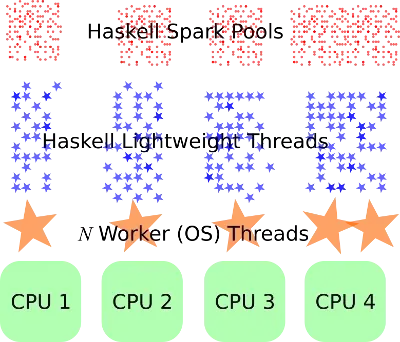