Please check the below steps for getting a trigger when the button in a WebView is clicked:
about_us.html
<html>
<head>
<script type="text/javascript">
function invokeNative() {
MessageInvoker.postMessage('Trigger from Javascript code');
}
</script> </head>
<body>
<form>
<input type="button" value="Click me!" onclick="invokeNative()" />
</form> </body>
</html>
- Added the code for loading WebView.
As per the below statement, you can see I am loading a WebView and
when I click on the button named Click me! in WebView the
JavascriptChannel in flutter will get invoked with a message "Trigger
from Javascript code"
import 'dart:convert';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:webview_flutter/webview_flutter.dart';
class WebViewApp extends StatefulWidget {
WebViewApp({Key key, this.title}) : super(key: key);
final String title;
@override
_WebViewAppState createState() => _WebViewAppState();
}
class _WebViewAppState extends State<WebViewApp> {
WebViewController _controller;
Future<void> loadHtmlFromAssets(String filename, controller) async {
String fileText = await rootBundle.loadString(filename);
controller.loadUrl(Uri.dataFromString(fileText,
mimeType: 'text/html', encoding: Encoding.getByName('utf-8'))
.toString());
}
Future<String> loadLocal() async {
return await rootBundle.loadString('assets/about_us.html');
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: FutureBuilder<String>(
future: loadLocal(),
builder: (context, snapshot) {
if (snapshot.hasData) {
return WebView(
initialUrl:
new Uri.dataFromString(snapshot.data, mimeType: 'text/html')
.toString(),
javascriptMode: JavascriptMode.unrestricted,
javascriptChannels: <JavascriptChannel>[
JavascriptChannel(
name: 'MessageInvoker',
onMessageReceived: (s) {
Scaffold.of(context).showSnackBar(SnackBar(
content: Text(s.message),
));
}),
].toSet(),
);
} else if (snapshot.hasError) {
return Text("${snapshot.error}");
}
return CircularProgressIndicator();
},
), // This trailing comma makes auto-formatting nicer for build methods.
);
}
}
As you can see in the code there is a JavascriptChannel
which will get invoked when the user clicks a button in webview. There is a key to identify the channel which in my case was MessageInvoker
.
Hopes this will do the trick...
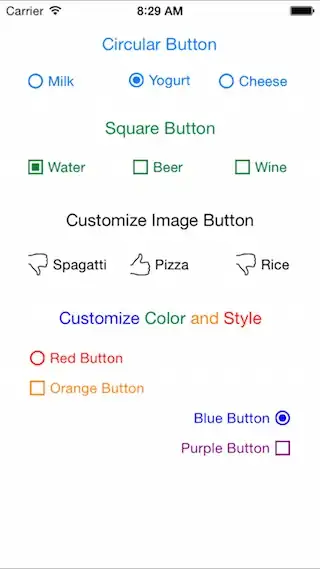