Plot lists and annotate
gender = ['M', 'F']
numbers = [1644, 1771]
plt.figure(figsize=(12, 6))
p = plt.bar(gender, numbers, width=0.1, bottom=None, align='center', data=None)
plt.bar_label(p)
plt.show()
Plot with pandas and annotate
- Convert the lists to a dataframe and plot with
pandas.DataFrame.plot
df = pd.DataFrame({'value': numbers, 'gender': gender})
ax = df.plot(x='gender', kind='bar', figsize=(12, 6), rot=0, legend=False, align='center', width=0.1)
ax.bar_label(ax.containers[0])
plt.show()
Original Answer
- In order to specify the horizontal alignment of the annotation, use the
ha
parameter
- As per the suggestion from JohanC
- A trick is to use
f'{value}\n'
as a string and the unmodified value
(or numbers
) as y position, together with va='center'
.
- This also works with
plt.text
. Alternatively, plt.annotation
accepts an offset in 'points' or in 'pixels'.
Option 1
- From
lists
of values & categories
import matplotlib.pyplot as plt
gender = ['M', 'F']
numbers = [1644, 1771]
plt.figure(figsize=(12, 6))
bars = plt.bar(gender, numbers, width=0.1, bottom=None, align='center', data=None)
for i in range(len(numbers)):
plt.annotate(f'{numbers[i]}\n', xy=(gender[i], numbers[i]), ha='center', va='center')
Option 2
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame({'value': [1771, 1644], 'gender': ['F', 'M']})
plt.figure(figsize=(12, 6))
bars = plt.bar(df.gender, df.value, width=0.1, bottom=None, align='center', data=None)
for idx, (value, gender) in df.iterrows():
plt.annotate(f'{value}\n', xy=(gender, value), ha='center', va='center')
Plot Output
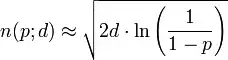